Welcome to the world of JUnit testing guide in Java! Be it a highly advanced or inexperienced developer, knowing JUnit for unit testing is very important to build a robust and bug-free software application.
In this tutorial, we are going to demystify unit testing using JUnit a test framework with huge popularity for its robust functionality and simplicity in usage.
By the end of this guide, you will have acquired the ability to do JUnit testing in your Java projects so that your software works perfectly in all conditions. Be prepared to increase your coding potential to benchmark applications.
Setting Up JUnit in Java
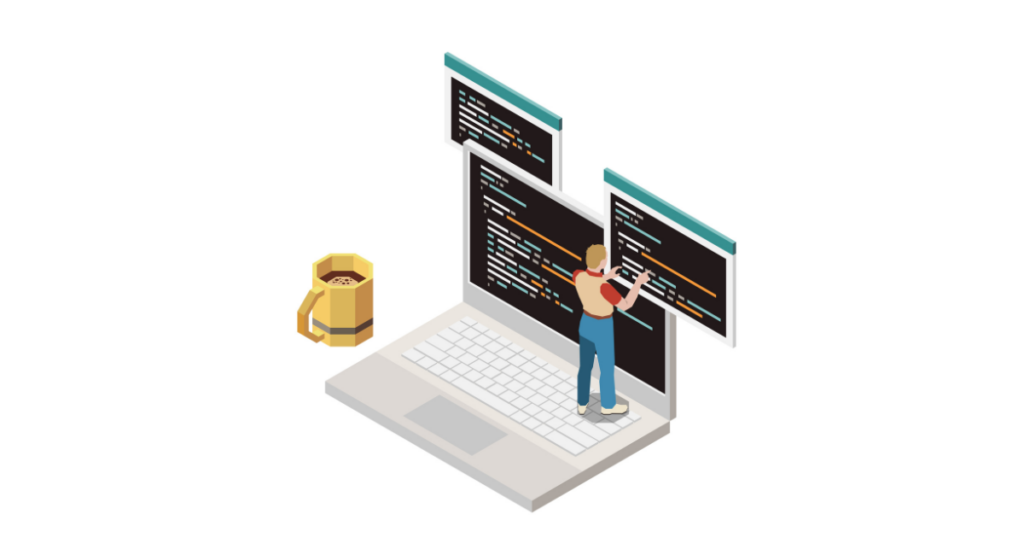
Installing JUnit
First of all, you are going to have to set up the library for JUnit in order to do any unit testing in Java. You can easily add JUnit as a dependency in your \`pom.xml\` file. Just append this dependency.
\`\`\`xml
junit
junit
4.13.2
test
\`\`\`
For Gradle users, add this line to your \`build.gradle\` file under dependencies:
\`\`\`groovy
testImplementation 'junit:junit:4.13.2'
\`\`\`
This will download and add the JUnit library to your project, enabling you to write and run unit tests.
Configuring JUnit in your Java project
After installation, configuring JUnit in your Java project requires setting up your development environment. If you are using an IDE like Eclipse or IntelliJ IDEA, these environments typically handle JUnit integration smoothly.
Once JUnit is added to your project, you should configure your IDE to recognize the \`test\` folder where your unit tests will reside.
Ensure that this folder is marked as a Test Source Root, so that the IDE knows to treat the files within as test files and execute them separately from your normal application code.
Writing Your First JUnit Test Case
Importing necessary libraries
To use JUnit, you need to import the necessary libraries in your test class. Typically, this would be at least the following imports:
\`\`\`java
import org.junit.Test;
import static org.junit.Assert.*;
\`\`\`
These imports allow you to use annotations to define test methods with \`@Test\`, and assertions like \`assertEquals\` to verify the expected results of your test.
Creating test methods
A JTest test method in Java is a public method annotated with \`@Test\`, and it contains the code to perform a specific test. Here's a simple example:
\`\`\`java
public class SampleTest {
@Test
public void testAddition() {
assertEquals(2, 1 + 1);
}
}
\`\`\`
Each test method typically tests a small unit of functionality, ensuring modularity and simplicity in testing.
Asserting expected results
The core of JUnit testing lies in assertions that verify the state of the test either passes or fails based on the expected outcomes. JUnit provides several assertion methods in the \`Assert\` class, like \`assertEquals\`, \`assertTrue\`, and \`assertNotNull\`.
All these methods apply to a different cause:
- \`assertEquals\` checks if two values are equal.
- \`assertTrue\` asserts that a condition is true.
- \`assertNotNull\` ensures an object is not null.
In case of test failure, JUnit sends a clear and concise message about which assertion is not met. It helps the developer to track the problem and fix efficiently.
Applying assertions effectively means you can be sure that your unit tests are robust and reliable.
Running JUnit Test Cases
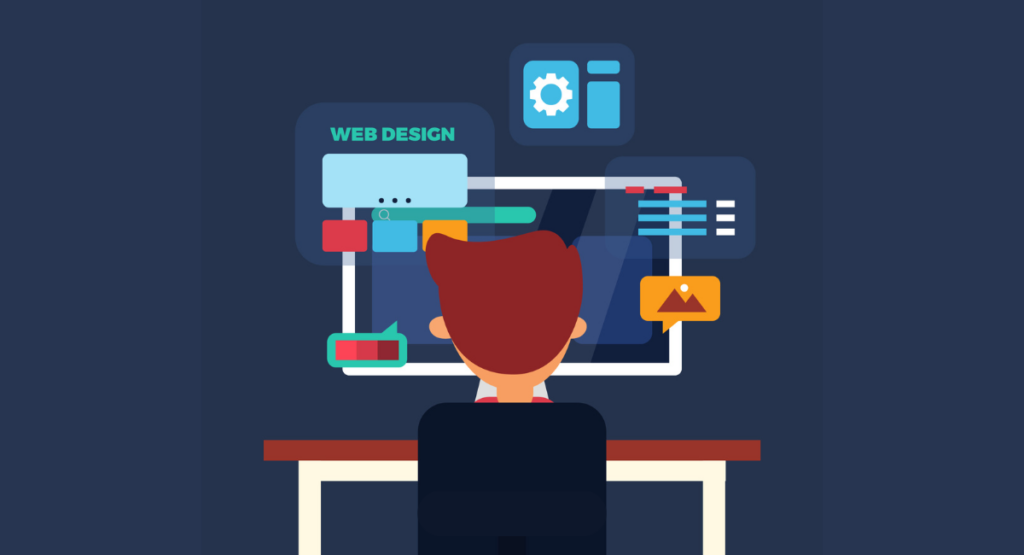
Running JUnit tests is both easy and straightforward in any Java development, since it may be either run from most Integrated Development Environments or directly from the command line. Each method has advantages and is better suited to different development scenarios.
Running tests from IDE
Running JUnit tests from within an IDE like Eclipse, IntelliJ IDEA, or NetBeans is pretty straightforward. They usually provide a specific pane where individual test cases are listed alongside their status and offer tools for running them one by one or as a whole.
In most IDEs, you would run a JUnit test by right-clicking on the test file and executing 'Run As – JUnit Test'.
Again, the IDE handles all the other details, compiling the tests, running them, and providing visual feedback about their results.
Running tests from command line
For those who prefer running tests from the command line or who simply need to automate these processes, several build tools like Maven or Gradle can be used.
With Maven, for example, you will use this by simply issuing the command mvn test in your project directory.
In scenarios like automation and continuous integration, this approach becomes very useful since developers can integrate test scripts as part of their build processes.
Understanding test results
Understanding the result of your JUnit tests is extremely critical for efficient debugging and delivery of robust software functionality.
JUnit makes test result messages very loud and clear in its output, usually including a success indicator, assertion failures, and a stack trace in case of failure.
It will display the green bar when everything has passed and a red bar when there are one or more failures, hence putting the developer in a better position to identify the problem quickly and fix it.
Best Practices for JUnit Testing in Java
Adopting best practices in JUnit testing not only helps in writing effective test cases but also enhances the maintainability and scalability of your test suite.
Test naming conventions
Consistency in naming your test methods is vital for maintainability and understanding.
It's generally recommended to start the name with ‘should’, followed by what the method does, and ending with the condition under which it does it, for example, \`shouldReturnTrueWhenDataIsValid()\`.
This practice helps in quickly identifying test purpose and conditions just by looking at its name.
Using annotations effectively
JUnit allows you to create logical and effective structuring of your tests by use of such annotations as \`@Test\`, \`@Before\`, \`@After\`, \`@BeforeClass\`, and \`@AfterClass\.
These annotations will definitely help you write less, but it will be more readable. For example, \`@Before\` and \`@After\` can set up and tear down common test data. This helps make sure each test is run in isolation.
Handling exceptions in/en tests
Testing exceptions properly proves that your application behaves correctly under error conditions. JUnit supports this with the\`@Test\` annotation using the \`expected\` attribute.
For example, \`@Test(expected = IndexOutOfBoundsException.class)\` will automatically pass if the test method throws the expected exception.
Not only does this make your tests more robust, but also much clearer in their intentions about what exceptions are handled in your application code.
Advanced Junit Features
Parameterized Tests
This may be useful in case scenarios where you want to run the same test several times on different inputs. Even redundancy of code can be harnessed with this feature in JUnit.
This cleans up the code and makes it more readable. You will replace the `@Test` with the \`@ParameterizedTest\` annotation and then pass arguments using \`@ValueSource\` or \`@CsvSource\`.
Test Suites
Test suites in JUnit are great for organizing tests and running them together as a batch. This can be especially helpful when you have tests that should be grouped for specific features or modules.
You can create a test suite by annotating a class with \`@RunWith(Suite.class)\` and \`@Suite.SuiteClasses({Test1.class, Test2.class})\`, thus specifying which tests to include in the suite.
Running a test suite executes all included tests, making it an excellent tool for comprehensive testing cycles.
Mocking with Mockito
Mockito is one of the most used mocking libraries, going along with JUnit to isolate test environments from external dependencies.
Very important in unit testing is mocking, since you would want to test only the component under test and not the functionality of some complex dependency.
Mockito provides you with simple annotations and methods such as `@Mock` and `when(…).thenReturn(…)` for creating mock objects and stubs. This is quite an essential technique to have correct, fast, and efficient tests when you deal with external systems or complex logic.
Book a Demo and experience ContextQA testing platform in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
JUnit has proved to be a fine tool for developers who want to ensure that their Java applications are robust and error-free.
In this tutorial, we have covered all the basics of how JUnit can be used for unit testing, starting from environment setup to writing and running tests.
Embracing JUNIT in your development process can make the development cycle smoother and faster by creating more reliable software, since it helps in detecting problems very early.
More important, though, is the fact that the success of a software project depends on proper and regular testing. Harness the power of JUnit to take your projects up to another level of competence, and keep on eyeing new features and updates.
Also Read - Understanding the Software Development Process