When you want to align an image using CSS, it might seem easy at first. But getting it perfectly centered can be trickier than you think. This guide is here to help you center images both horizontally and vertically in different HTML situations.
Whether you're working on a personal blog, a business site, or an app, mastering the art of image centering in CSS can really spruce up how your web pages look and feel.
With simple explanations and examples, this guide is a great tool for newbies and seasoned front-end developers wanting to level up their layout game.
Understanding Centering an Image in CSS
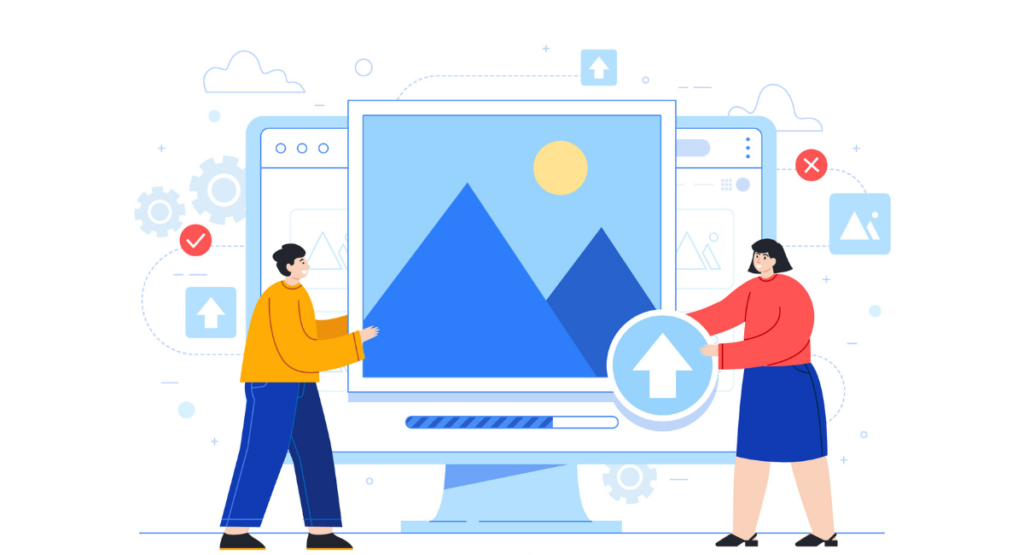
The Importance of Centering Images
Nailing that centered image look isn't just a nice-to-have for websites – it's a game-changer. When those visuals are perfectly positioned, the whole layout just clicks.
It's like the web design equivalent of a well-choreographed dance routine. Everything falls into harmony, and your eyes can't help but follow the flow.
But centering images isn't just about making things pretty (although that's a huge perk). It's also a power move for creating a top-notch user experience.
Especially on sites built to adapt to different screen sizes, proper alignment ensures your content always looks crisp and intentional, whether someone's browsing on a massive desktop or a pocket-sized mobile.
Different Methods for Image Centering in CSS
CSS provides a range of techniques for centering an image, each suitable for different scenarios and needs. Grasping these methods empowers developers to choose the most efficient strategy based on their project's context.
Common approaches involve utilizing the \`text-align\` property on a container, applying the \`margin\` property directly to the image, or making use of contemporary CSS layouts such as Flexbox or Grid.
Each method boasts unique advantages and can stand alone or be combined with others to attain the desired alignment and responsiveness.
Horizontal Image Centering Techniques
Using text-align Property
One of the simplest ways to center an image horizontally is by using the \`text-align\` property on the image's container. This method is particularly effective when the image is displayed as an inline element (which is default for images):
\`\`\`css
.container {
text-align: center;
}
\`\`\`
By setting \`text-align: center;\` on the container, all inline content inside the container, including images, will be centered. This approach is straightforward and works well for simple layouts where no other alignment adjustments are needed.
\`margin\`
Another commonly used technique involves the \`margin\` property. By setting the margins to auto, you can center an image inside a block-level container:
\`\`\`css
img {
display: block;
margin-left: auto;
margin-right: auto;
}
\`\`\`
This method centers the image by evenly distributing the margins on both sides. It requires the image to be set as a block element (\`display: block;\`), as inline elements do not respect horizontal margin settings.
Using Flexbox
Flexbox provides a more powerful and adaptable method for centering components, including images. To center an image horizontally with Flexbox, apply the following settings to the container:
\`\`\`css
.container {
display: flex;
justify-content: center;
}
\`\`\`
In this configuration, the Flexbox layout model is utilized to align the children (specifically, the image) at the center of the container along the main axis (from side to side).
Flexbox proves to be highly beneficial for intricate layouts and scenarios where you aim to center numerous items or attain vertical centering, which we'll cover in more detail later.
Vertical Image Centering Techniques
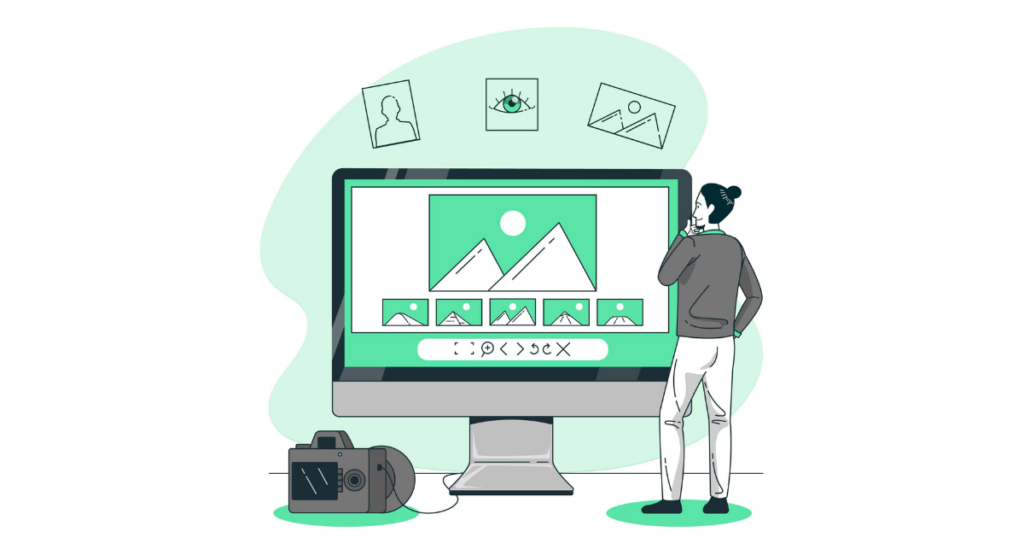
Using Line Height
Among the most straightforward methods to vertically center an image, especially when it's within container with text, involves employing the CSS \`line-height\` property.
This method is simple yet most effective when the container does not contain multiple lines of text. Firstly, adjust the \`line-height\` property to correspond with the height of the container.
Then, make sure that the image is showcased as an inline-block element using \`display: inline-block;\`. Here's an example:
\`\`\`css
.container {
height: 200px;
line-height: 200px;
text-align: center;
}
.img {
vertical-align: middle;
}
\`\`\`
In this CSS, the \`.container\` holds the image and possibly a single line of text, both aligned vertically and horizontally.
Using Flexbox
Flexbox is so cool! It helps to center things vertically and can hold a lot of goods in a box. To center an image vertically in Flexbox, add '\display: flex\' to the box, followed by '\align-items: center\' to have those objects aligned up properly. Here's how to accomplish it.
\`\`\`css
.container {
display: flex;
align-items: center;
justify-content: center; / This also centers horizontally /
height: 200px;
}
\`\`\`
This method centers the image perfectly within the container, regardless of the height or multiple texts or elements inside the container.
Using Grid
CSS Grid is another powerful layout tool that simplifies alignment challenges, including vertical centering. To center an image vertically using Grid, assign `\display: grid;\` to the container and use `\align-items: center;\` for vertical alignment:
\`\`\`css
.container {
display: grid;
align-items: center;
height: 200px;
}
\`\`\`
This setup ensures that the image remains centered, even as you adjust the container's dimensions or add more content.
Centering Image in Both Horizontal and Vertical Directions
Using Flexbox
To properly position an image in the center both horizontally and vertically using Flexbox, you need to blend the technique of vertical alignment with horizontal centering.
Begin by setting your container to \`display: flex;\`, After that, specify \`align-items: center;\` for vertical alignment and \`justify-content: center;\` for horizontal alignment.
\`\`\`css
.container {
display: flex;
align-items: center;
justify-content: center;
height: 200px;
}
\`\`\`
This code snippet makes Flexbox a robust solution for perfectly centering items within any container.
Using Transform Property
The transform property offers a more explicit approach when you need to center an image exactly within a container. First, position the image absolutely within its relative container. Then, use the \`transform\` property to adjust the positioning:
\`\`\`css
.container {
position: relative;
height: 200px;
}
.img {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
\`\`\`
This hack properly aligns the image by nudging it down 50% and above 50%, then pulling it back by half its size. It works like magic on boxes of all shapes and sizes, maintaining the image dead center regardless.
Tips for Responsive Image Centering
When creating websites that perform on all devices, it's critical that images play well. CSS to center images not only improves the appearance of your website, but it also makes it easier to navigate. Below are some tips to ensure your images remain centered and responsive:
Use Percentages Rather than Fixed Dimensions
To maintain responsiveness, avoid using fixed width and height for images. Instead, use percentages. Setting the width as a percentage allows the image to adapt based regionally on the size of its container.
Typically, setting the width to a percentage while leaving the height auto will maintain the aspect ratio of the image:
\`\`\`css
img {
width: 50%;
height: auto;
display: block;
margin: 0 auto;
}
\`\`\`
Consider the Display Property
The display property plays a pivotal role in how an element is viewed across different devices. For centering images, setting the display property to 'block' is essential because inline elements (the default for images) do not respond to top and bottom margins. This small adjustment enables the use of 'margin: auto':
\`\`\`css
img {
display: block;
margin: 0 auto;
}
\`\`\`
Media Queries for Enhanced Flexibility
To accommodate various devices, utilize media queries to alter image styles at different breakpoints. This approach ensures that the image remains centered and visually consistent, regardless of the device. For example:
\`\`\`css
@media (max-width: 768px) {
img {
width: 100%;
height: auto;
}
}
\`\`\`
By testing and adjusting the CSS properties with these tips, you can ensure that images on your website are effectively centered and responsive across all viewing platforms.
Book a Demo and experience ContextQA testing platform in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
Centering images in CSS is a fundamental skill that enhances the aesthetic appeal and functionality of web pages. By mastering tricks like Flexbox, Grids, and old-school margins, designers have all they need to make sure images sit just right in any layout.
These skills don't just make your content pop but also give visitors a top-notch experience on your site. So whether you're just starting out or been around the block, honing these skills will help you make awesome-looking websites that everyone loves.
Also Read - Best Android Emulator for Linux – Updated Edition