CSS Selectors in Selenium WebDriver are very powerful and allow a tester or a developer to efficiently interact with Web elements. They identify and locate elements within a web page by matching a pattern against the structure and attributes of the HTML.
The understanding of CSS Selectors can greatly help in easing the automation process by providing a very fine-grained way for targeting and manipulation of elements for web testing.
The following is a step-by-step guide on how to use CSS Selectors for improving the accuracy of your automation scripts.
Understanding CSS Selectors
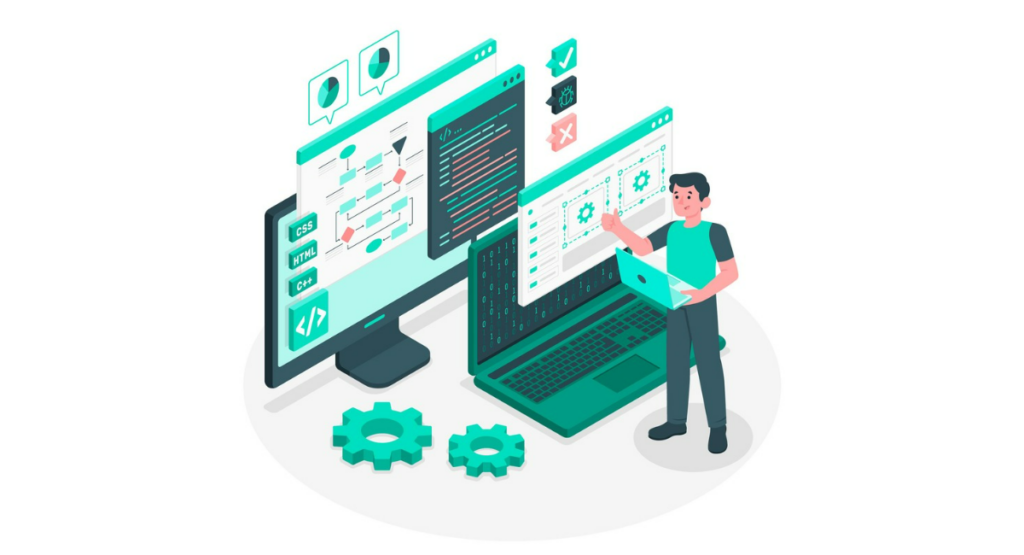
What are CSS Selectors?
There could be different attributes from which elements of a web page can be selected. They may include an ID, class, type, or by its relationship to other elements.
The following selectors are among the cornerstones of Cascading Style Sheets and are used for applying style on a particular element that corresponds to the pattern given by the selector.
CSS selectors are also used when locating and manipulation of specific elements in the DOM of a webpage are required with the open source automation tool Selenium WebDriver.
Importance of CSS Selectors in Selenium
Within Selenium WebDriver, CSS selectors play a core role in automation for any web-based application. This is used to locate all those elements on the webpage with which a user may need to interact, like input fields, buttons, and dropdown menus.
Precisely selected elements using CSS can enhance test script performance. They also provide a flexible and short way for element identification compared to other identifiers, specifically XPath, in a complex web application.
Furthermore, CSS selectors are faster in performance and supported by every modern browser due to which they are given more preference by developers and testers.
Types of CSS Selectors
ID Selector
The ID selector chooses one element; it does this based on the id attribute of an HTML element. One of the easiest and most effective methods of choosing elements using CSS is the ID selector, which is represented by a hash (#) followed by the value of an 'id' attribute.
The idea is that the per-page-element equivalent ID selector is very unique and thus fast and reliable while searching for elements in a page. For example, "#login-button" will become the CSS selector of an element that has its 'id' set to "login-button".
Class Selector
The Class selector targets HTML elements that have a specific class attribute. It is represented by a dot (.) followed by the class name.
Class selectors are useful when multiple elements share the same class and styles or interactions need to be applied uniformly. For instance, the CSS selector to find all elements with the class "highlight" would be ".highlight".
Attribute Selector
Attribute selectors in CSS are used to select elements based on the presence or value of a given attribute. These selectors are powerful because they offer fine-grained control over element selection.
Syntax involves using square brackets around the attribute, sometimes including a value. For example, selecting all input elements where the type attribute equals "text" would be written as "input[type='text']".
Combining Selectors
Combining CSS selectors provides an even greater level of specificity and control, allowing the selection of elements that fit multiple criteria. There are several methods to combine selectors in CSS:
- Descendant selector: Spaces between selectors (e.g., ".menu li" selects all
- elements inside elements with class "menu").
- Child selector: The greater-than sign (>) selects direct children (e.g., "div > p" selects all
elements that are direct children of
elements).
- Adjacent sibling selector: The plus sign (+) selects an element immediately following another (e.g., "div + p" selects the first
that directly follows a
).
By mastering these CSS selectors, Selenium testers can optimize their scripts to interact with web elements efficiently and accurately.
Using CSS Selectors in Selenium
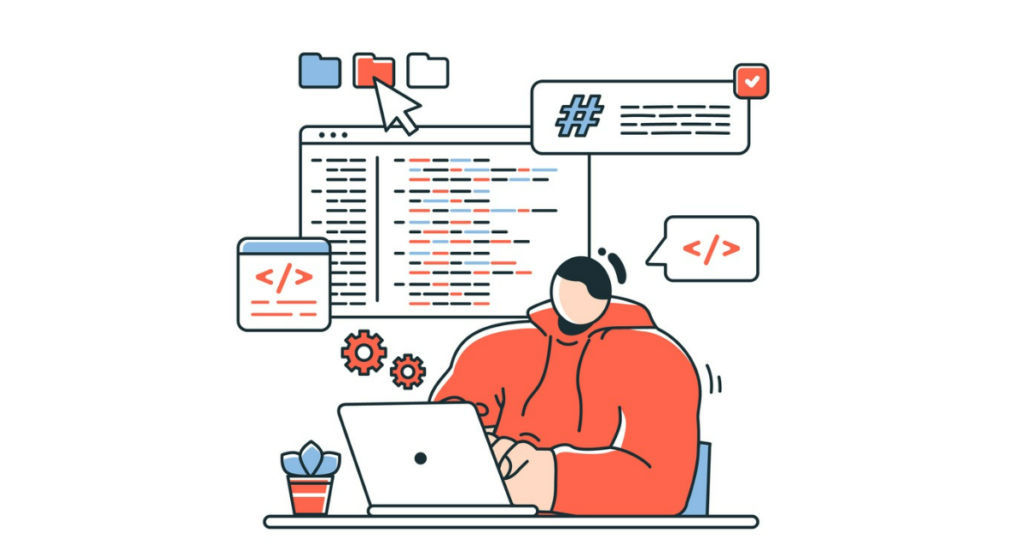
Locating Elements by ID
In Selenium WebDriver, locating web elements by ID is highly efficient due to the uniqueness of the ID attribute in HTML. You can use CSS selectors for this purpose by prefixing the ID value with a hash (#) symbol.
For example, if an element is defined in HTML as , you can locate this element using the CSS selector "#username". This method is recommended when you know the exact ID of the element, ensuring quick and precise element selection.
Locating Elements by Class
To locate elements by their class attributes, you use the period (.) symbol followed by the class name. For instance, if an HTML element is marked with the class attribute as
, it can be accessed in Selenium using the CSS selector ".container". Remember that classes are not unique, so this selector might locate multiple elements. In such cases, further specificity might be needed either by chaining more conditions or by using other element attributes.
Locating Elements by Attributes
Using CSS selectors, one could find elements based on any attribute. Now, it's not limited only to the 'id' or 'class' attributes. It does so by enclosing the attribute name with the value in square brackets [].
For example, if somebody is looking for 'input' elements that have a "name" attribute with the value "email", they should then use 'input[name="email"]'.
This approach is actually very flexible and can be further applied to any element, by attribute, like 'href', 'title', 'type', etc.
Locating Elements by Combining Selectors
Combining selectors can significantly enhance your ability to locate elements with precision. For example, to find a button within a specific section that has both an ID and a class, you might use a selector like "#main .button".
This selector locates elements with the class "button" inside an element with the ID "main". Combining selectors helps in narrowing down the search and targeting elements in complex DOM structures.
Practical Examples of Using CSS Selectors
Example 1: Login Form
Consider a login form with fields for username and password. Suppose the HTML is structured with an ID for the form and classes for the inputs.
The CSS selectors used might be "#loginForm .username" and "#loginForm .password". Here’s how you would implement them in Selenium WebDriver to interact with these fields:
\`\`\`java
WebDriver driver = new ChromeDriver();
driver.get("http://example.com/login");
WebElement username = driver.findElement(By.cssSelector("#loginForm .username"));
WebElement password = driver.findElement(By.cssSelector("#loginForm .password"));
username.sendKeys("your_username");
password.sendKeys("your_password");
\`\`\`
This snippet efficiently locates the username and password fields within the login form and inputs values into them.
Example 2: Navigation Menu
Let’s say you want to interact with a navigation menu where each item has a class "nav-item" and is contained within a div with ID "navbar".
To click on a specific menu item, such as "Home", you could use a combined selector like "#navbar .nav-item:first-child". Here's how it might look in your Selenium script:
\`\`\`java
WebElement homeMenu = driver.findElement(By.cssSelector("#navbar .nav-item:first-child"));
homeMenu.click();
\`\`\`
This code snippet will effectively locate and interact with the "Home" item in the navigation menu. These practical examples illustrate how using CSS selectors in Selenium can simplify interacting with complex web page elements.
Best Practices for Using CSS Selectors in Selenium
Selecting Stable and Unique Selectors
When using CSS Selectors in Selenium, it’s crucial to choose identifiers that are both stable and unique. Stable selectors remain consistent even when the design or layout of the application undergoes minor changes.
Unique selectors, on the other hand, refer to those identifiers exclusive to a specific element, ensuring that the automation does not interact with the wrong element.
Use attributes like id, name, or class with specific values to maintain a reliable selection path. Moreover, incorporating attributes that directly relate to the element’s functionality, like “name=’submit-button’,” helps enhance the robustness of the test scripts.
Avoiding Fragile Selectors
Selectors that depend heavily on the structure of the webpage can become fragile, causing tests to fail when developers modify the UI. To avoid this, refrain from using complex XPath expressions that rely on multiple layers of parent-child relationships.
Instead, focus on simpler CSS selectors that target elements based on their immediate attributes and classes without chaining too many conditions. This simplicity ensures that the scripts are less likely to break over minor UI updates.
Handling Dynamic Elements
Web elements that change dynamically with page loads, user interactions, or time factors pose a significant challenge in automated testing.
Use CSS selectors that are capable of identifying these elements reliably across different states. For example:
- Attribute selectors like [attribute*='value'] can match elements containing specific patterns.
- Using pseudo-classes such as :nth-child() can help in selecting elements that appear in a repeat structure but might have identifiers that change dynamically.
In summary, selecting efficient and precise CSS selectors in Selenium not only ensures more reliable automated tests but also reduces maintenance efforts by minimizing the need for frequent updates to the test scripts due to changes in the UI.
Book a Demo and experience ContextQA testing platform in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
In the realm of Selenium WebDriver, CSS selectors stand out as potent and flexible tools for pinpointing specific web elements essential for successful test automation.
Mastering their use can significantly improve the efficiency and reliability of your tests. By leveraging the examples and techniques covered, you can hone your skills in creating precise, robust tests that are less susceptible to changes in web UI.
Remember to practice regularly, as proficiency in CSS selector usage is a critical component of effective web automation.
Also Read - Agile Development Methodologies: An Essential Guide