While working with web testing using Selenium WebDriver, it becomes very important to know how the mechanism of the wait commands is.
And these commands deal with loading time and variability in network latency in your application so that your automated tests become reliable in execution.
Selenium provides several types of wait mechanisms implicit, explicit, and fluent wait. Each type serves unique purposes and can crucially impact the efficacy of your test scripts.
Throughout this blog, we will delve into each wait command, explore their uses, and guide you on when to employ them in your Selenium scripts. Understanding these commands will enable you to optimize testing scripts effectively and ensure accurate automation.
Implicit Wait
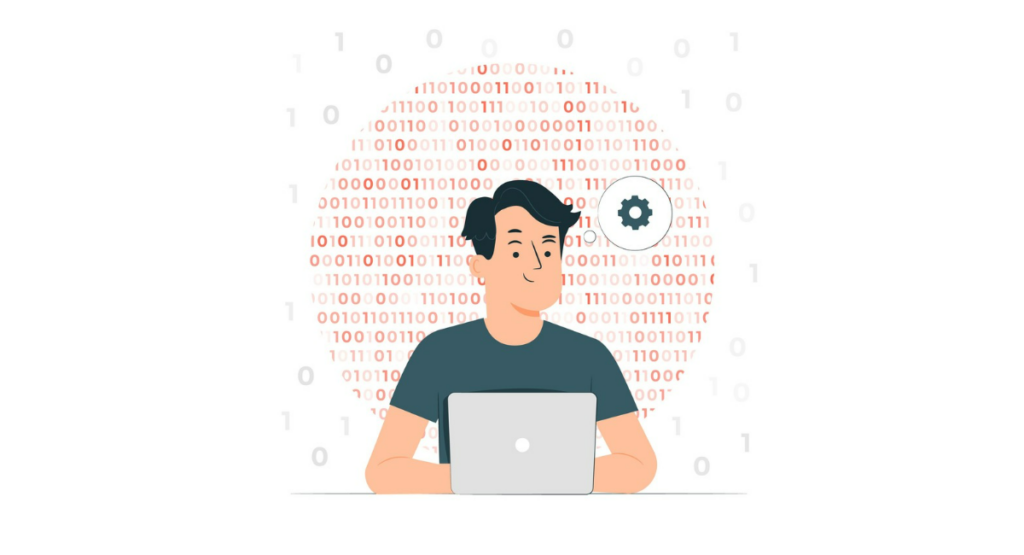
Definition and Purpose
Implicit wait in Selenium is the technique of commanding the webdriver to speak momentarily for a specified time before it gives up on seeking or finding an element on the page that isn't instantly available.
Implicit waits are mostly used to define the time the WebDriver will poll the DOM before returning while searching for any element (or elements) not immediately visible.
This is of importance in so many cases where an element in the web page may take some milliseconds to load as a result of network latency or some other JavaScript operations.
Implementation in Selenium
Implementing implicit wait in Selenium is straightforward. You need to set the wait mechanism once per session, typically right after initiating the web driver.
For example, in Python, you can set an implicit wait using the following line of code:
\`\`\`python
driver.implicitly_wait(10) # waits up to 10 seconds
\`\`\`
This line directs Selenium to wait up to 10 seconds before throwing a NoSuchElementException if it cannot find the desired elements within the given time frame.
It’s important to note that once set, the implicit wait is set for the life of the web driver object instance.
Explicit Wait
Definition and Purpose
Explicit wait, unlike implicit wait, is used to halt the execution until a specific condition occurs or the maximum time has elapsed.
Explicit waits are confined to a particular element with specific conditions, making them more suitable for handling scenarios where certain elements may take variable time to load.
Additionally, explicit wait can also be used for non-element conditions such as waiting for a page to load or a file to be available to download.
How to use in Selenium
To configure an explicit wait in Selenium, you would generally employ WebDriverWait in conjunction with ExpectedConditions. Here's an example in Python to demonstrate its usage:
\`\`\`python
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.WebDriver.support import expected_conditions as EC
driver = WebDriverWait(driver, 10)
element = wait.until(EC.visibilityofelement_located((By.ID, 'example')))
\`\`\`
In this snippet, the WebDriver will wait up to 10 seconds for an element matching the ID "example" to become visible on the page.
Comparison with Implicit Wait
While both waits are useful for improving the reliability of a Selenium script, they serve slightly different purposes and behave differently:
- Implicit Wait: Sets a default waiting time throughout the lifetime of the WebDriver, applicable to all elements.
- Explicit Wait: Applies a wait for a particular instance only, with specific conditions.
Unlike implicit wait, explicit wait provides a more nuanced capability to handle different loading times and is only initiated when used making it more flexible for dynamic content where wait times might differ substantially from one element to another.
This specificity also means explicit waits can lead to more maintainable test scripts, as they adapt to varied network conditions and page load times.
Fluent Wait
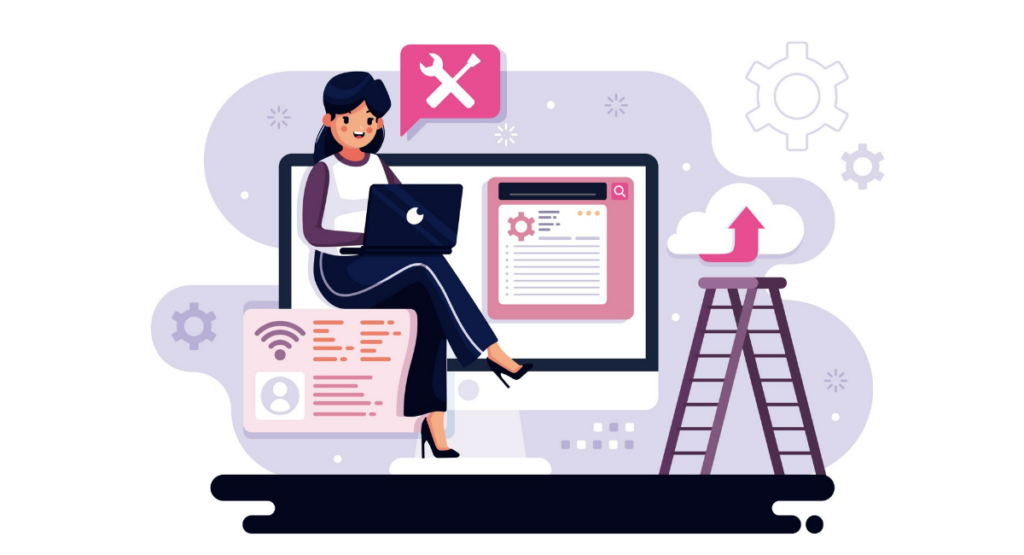
What is Fluent Wait
Fluent Wait in Selenium is a type of Conditional Wait where it can define the maximum amount of time to wait for a condition, as well as the frequency with which to check the condition before throwing an "ElementNotVisibleException".
Fluent Wait works by setting the maximum wait time and polling frequency, then ignoring instances of specified exceptions until the waited element becomes available on the page or the timeout expires.
This is useful in cases where elements are loaded at different intervals or require more complex conditions for visibility.
How it Differs from Implicit and Explicit Wait
Fluent Wait is often considered more powerful and flexible than both Implicit and Explicit Waits due to several distinctive characteristics. The main difference is the ability to define the frequency of checks performed to determine whether a condition is met or not.
In contrast, Implicit Wait sets a fixed wait time for the entire duration before throwing an exception, thereby potentially waiting longer than necessary.
On the other hand, Explicit Wait will check for the element at regular intervals until it finds it or times out, but doesn’t allow for the frequency of checks to be adjusted.
Fluent Wait, however, permits both maximum wait time and the polling frequency to be customized, thus providing finer control over the wait mechanism.
Benefits of Using Different Selenium Wait Commands
Improving Test Stability
One major benefit of using different Selenium Wait commands is the enhancement of test stability. By effectively utilizing Implicit, Explicit, and Fluent Waits, testers can ensure that the application is ready for interaction before executing the next steps.
This helps to reduce test failures caused by elements that are not yet available or loaded on the web page, hence minimizing false negatives and providing a more robust testing framework.
Enhancing Script Reliability
Incorporating multiple wait strategies in test scripts significantly enhances their reliability. Scripts are less likely to fail unexpectedly when waits are properly implemented according to the situation.
For instance, using Fluent Wait allows a more tailored approach where tests wait just enough for elements to be interactable, but not longer than necessary, thus optimizing the performance and accuracy of test executions.
This nuanced approach helps in building more reliable and efficient testing scripts that perform well even under varying network conditions or with dynamic web contents.
Best Practices for Using Selenium Wait Commands
Using Selenium wait commands effectively is crucial for creating reliable and efficient test scripts. Implementing best practices not only enhances the performance of tests but also ensures more accurate results by reducing flakiness caused by synchronization issues.
Tips for optimizing wait times
Following are some of the strategies for optimizing wait times in Selenium:
- Explicit Waits Over Implicit Waits: Explicit waits have conditions that will keep them holding until the criteria have been met and thus can be flexible and efficient in certain scenarios.
- Modify Timeouts Based on Network Conditions: In case your application is used with highly varying network conditions, adjust timeout settings accordingly to accommodate the load time.
-Apply Fluent Wait for More Dynamic Situations: Through Fluent Wait, it's possible to define both the maximum amount of time to wait for a condition and the frequency at which Fluent Wait needs to check if the condition is met.
That is applicable in situations where certain elements have a very uncertain loading time.
Common pitfalls to avoid
When utilizing Selenium wait commands, be conscious of the following pitfalls:
- Avoid using excessive fixed waits (Thread.sleep): This method can significantly slow down test execution times and lead to inefficient testing processes.
- Do not mix Implicit and Explicit waits: Using both can cause unpredictable wait times and can make debugging difficult. Stick to one type of wait strategy per test case.
- Ignoring exceptions in wait commands: Always ensure that your wait commands are set to handle exceptions appropriately. Not doing so can result in unhandled exceptions and test failures.
By adhering to these best practices and avoiding common mistakes, you can maximize the effectiveness and reliability of your Selenium test scripts.
Book a Demo and experience ContextQA testing tool in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
Implementing the right Selenium wait commands can markedly improve the stability and reliability of your automated testing scripts.
By choosing between implicit, explicit, and fluent waits based on the specific conditions and requirements of your test cases, you can ensure that your scripts are both efficient and robust.
Remember, while implicit waits provide a simpler, less flexible approach, explicit and fluent waits offer greater control with conditions-based management, unlocking more powerful and precise testing capabilities.
Thoroughly grasoming and utilizing these tools will undoubtedly elevate your testing processes and contribute significantly to the success of your automation projects.
Also Read - CSS Selector in Selenium: Locate Elements with Examples
We make it easy to get started with the ContextQA tool: Start Free Trial.