Welcome to our practical Selenium Webdriver tutorial in Java! If you're looking to pursue the arena of Automation Testing, then this certainly is the correct platform to be on.
Selenium Webdriver is an excellent tool for automating web browsers, thus helping one reproduce user activities to the last pixel. We will cover everything from setup of the environment to writing your very first test to mastering advanced features in this tutorial.
Whether you are totally new to the field of software testing or a seasoned developer who wants to add another tool in your toolbox, these practical examples will make you proficient in using Selenium Webdriver with Java.
Get ready to enhance your testing capabilities and drive efficiency in your software development processes.
Getting Started with Selenium Webdriver
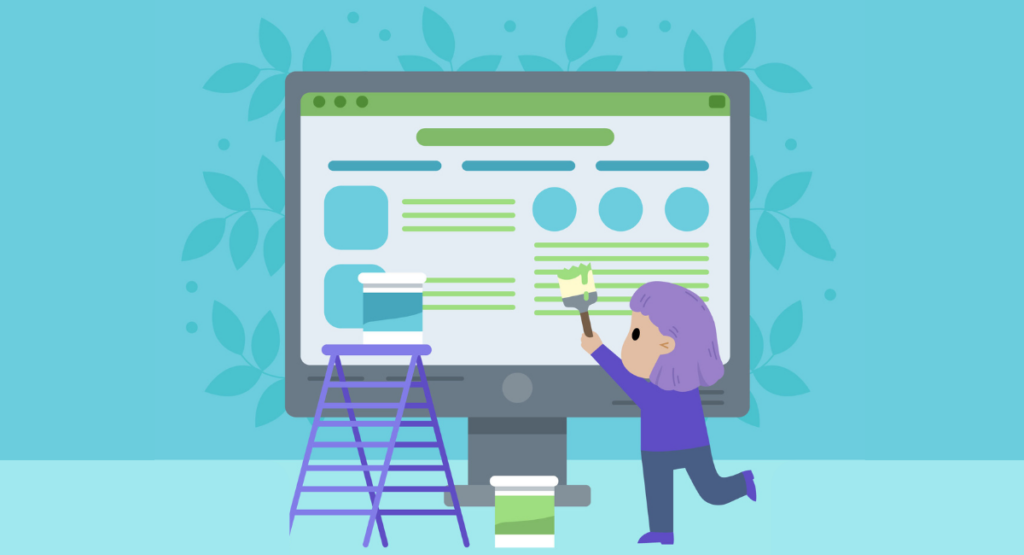
Setting up Selenium in Java
First and foremost, you have to prepare your Java development environment. After installing Java itself that is, the JDK plus an IDE like Eclipse, IntelliJ IDEA, or Visual Studio Code, you are good to go for setting up Selenium.
The Selenium Java client driver can be downloaded from the Selenium website. Once downloaded, add the Selenium JAR files to your project.
These JAR files can be included in your IDE by configuring them as dependencies in the project settings. Additionally, WebDriver requires drivers specific to each browser.
For instance, Chrome requires the ChromeDriver, and Firefox requires the GeckoDriver. Download and set the path to these drivers in your system environment or directly in your code.
Understanding the basics of Selenium Webdriver
Selenium Webdriver is one of the powerful tools for controlling web browsers programmatically and performing Browser Automation. It shall be compatible with various browsers such as Chrome, Firefox, and Internet Explorer.
The basic Selenium test script in Java has a few key steps: initialization of the WebDriver class, launching a browser, loading the webpage, and performing all operations like clicking and typing.
It is in the programmability of the interactions with web page elements and automation of tasks in fact, mimicking actions taken by users whereby WebDriver comes into its own as a power tool.
Such operations will help in creating automated, robust tests that can simulate user behavior quite accurately.
Locators in Selenium Webdriver
Using ID, Class, Name, Tag Name locators
In Selenium, locators enable the WebDriver to find and manipulate web page elements. The simplest and most reliable locators are ID, Class, Name, and Tag Name. An element’s ID is unique within a page, making it a straightforward choice for quick and precise element selection.
Class names and tag names can be used when multiple elements have the same structure, but varying content. The Name locator works similarly to ID but is not guaranteed to be unique, which can lead to multiple elements being identified.
A typical usage scenario might involve locating a text box by its ID and entering text, selecting a button by its name, and clicking it. Here’s a concise example:
- Using ID: \`WebElement signInButton = driver.findElement(By.id("signIn"));\`
- Using Class: \`List buttons = driver.findElements(By.className("buttonClass"));\`
- Using Name: \`WebElement firstNameInput = driver.findElement(By.name("firstName"));\`
- HTTP Using Tag Name: \`List links = driver.findElements(By.tagName("a"));\`
Exploring XPath and CSS locators
Advanced locators such as XPath and CSS selectors provide powerful ways to navigate through complex web pages. XPath uses paths to navigate through the HTML structure of a webpage, very much like a path in a traditional file system.
It is extremely versatile and can locate nested elements, and elements by condition, among other capabilities. CSS selectors identify elements based on their style attributes, and like XPath, can be used to pinpoint elements nested deep within a webpage’s DOM.
Both XPath and CSS selectors are indispensable in scenarios where simple locators like ID or Class are insufficient. Examples of using these locators include:
- XPath: \`WebElement loginButton = driver.findElement(By.xpath("//button[text()='Login']"));\`
- CSS: \`WebElement logoImage = driver.findElement(By.cssSelector("img.logo"));\`
These advanced locators offer enhanced flexibility and precision in scenarios where elements might not have unique IDs or classes.
Interacting with Web Elements
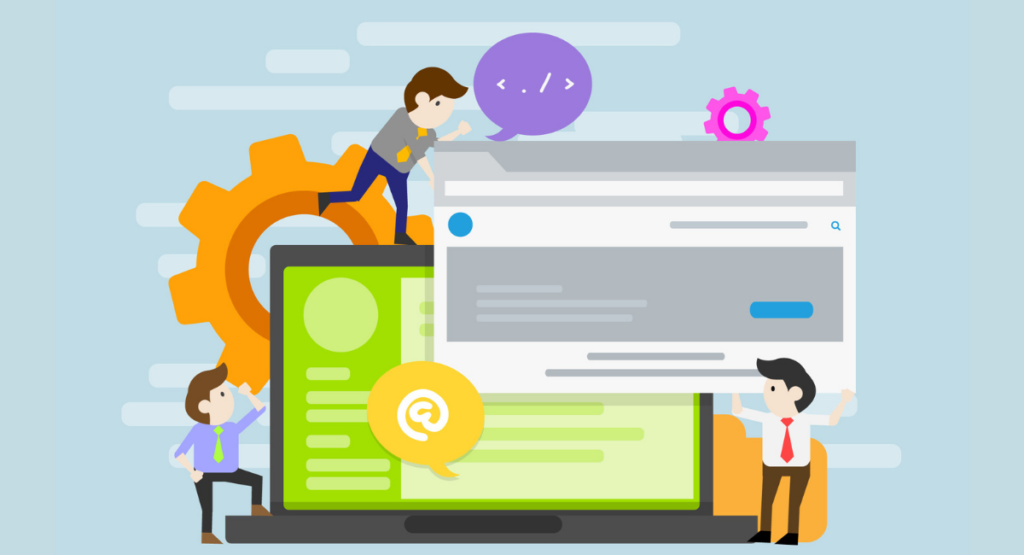
Interacting effectively with web elements is a fundamental part of using Selenium Webdriver. Web elements are the building blocks of web pages, and learning how to manipulate them is essential for successful automation testing.
Handling different types of web elements
Web pages are composed of various types of elements, including text boxes, buttons, drop-down menus, and even more advanced elements like sliders and date pickers. To deal with such elements, there are several available methods in Selenium, particularly:
- Text Boxes: You would first need to locate the element in use; to type in it, do something like \`driver.findElement(By.id("inputID")).sendKeys("Your text here");\`
- Buttons: Clicking a button is done by the \`click()\` method, e.g., \`driver.findElement(By.id("buttonID")).click();\`
- Checkboxes & Radio Buttons: You will select a checkbox or radio button using the very same \`click()\`` method.
Performing actions like click, type, select
Executing actions on web elements mostly revolves around the methods provided by the Selenium \`WebElement\` interface. Actions such as clicking (\`click()\`), typing (\`sendKeys()\`), and selecting from a drop-down (\`Select\` class) are straightforward.
Additionally, more complex sequences of actions can be performed using the Actions class, which enables you to build a sequence of actions using the builder pattern.
Wait Mechanisms in Selenium Webdriver
A common issue you might face when automating tests is the varying response time of web pages. Elements might load at different speeds, which makes it necessary to use waits. Selenium provides several wait mechanisms to handle these scenarios.
Implicit vs. Explicit Waits
Implicit waits are used to set up a default waiting time (say, 30 seconds) in your tests before Selenium throws a \`NoSuchElementException\`.
This method tells the driver to poll the DOM for a certain amount of time when trying to find any element (or elements) not immediately available. On the other hand, explicit waits are used to halt the execution until a particular condition is met.
They can be customized to wait for specific elements, making them more flexible. The \`WebDriverWait\` class along with \`ExpectedConditions\` helps in implementing explicit waits effectively.
Implementing fluent wait in Selenium
Fluent wait in Selenium is another sophisticated waiting mechanism where the frequency with which \`WebDriver\` checks the condition can be defined, along with the total amount of wait time.
It allows you to configure it to ignore specific types of exceptions while waiting for a condition, ensuring robust execution of test scripts.
Implementing it involves creating an instance of \`FluentWait\` class, setting the maximum wait time, the polling frequency, and the exception to ignore; and finally defining the condition to wait for using \`wait.until()\` method.
This approach is particularly useful when dealing with elements which might appear after different time intervals.
Working with Frames and Windows
Handling frames and multiple browser windows is an essential part of automating tests for complex websites. These techniques allow you to interact with elements that are not immediately accessible due to their placement in different sections of the website interface or in entirely separate windows.
Switching between frames
A frame is a part of a web page or browser window that displays content independent of its container. This content has its own HTML document, making it tricky to manage without special commands.
Selenium handles this by using the \`switchTo()\` method:
1. Identify the frame: You can select a frame using identifiers like name, ID, or index.
2. Switch to the frame: Use \`driver.switchTo().frame()\` method to switch control to the specified frame.
3. Interact with the frame: Now that you're in the context of the frame, you can interact with its elements.
4. Switch back to the main page: Once your tasks within the frame are complete, use \`driver.switchTo().defaultContent()\` to move back to the main document.
This process allows your test scripts to seamlessly interact with multiple frames on a page.
Managing multiple browser windows
Selenium WebDriver can also handle cases where interactions open new browser windows or tabs. You manage these scenarios with the following approach:
1. Get the handle of the current window: \`String parentWindowHandle = driver.getWindowHandle();\`
2. Perform actions that open a new window.
3. Switch to the new window: Use \`driver.switchTo().window(newWindowHandle);\` where \`newWindowDestHandle\` is the handle of the newly opened window.
4. Close the new window and switch back: After your tasks are complete, close it using \`driver.close();\` and switch back to the parent window using \`driver.switchTo().window(parentWindowHandle);\`.
Managing multiple windows is often used for testing scenarios such as authentication flows, file downloads, or external links.
Handling Alerts and Pop-ups
Web applications frequently use alerts and pop-ups to interact with users, and testing these features is crucial for end-to-end automation.
Dealing with alerts using Selenium
Alerts in web applications are simple but crucial user interfaces for interacting with users. Selenium Webdriver provides methods to interact with these alerts in a straightforward manner:
1. Wait for the alert to be present: This can be achieved using expected conditions.
2. Switch to the alert: \`Alert alert = driver.switchTo().alert();\`
3. Accept or dismiss the alert: Use \`alert.accept();\` to accept or \`alert.dismiss();\` to dismiss the alert. If the alert requires text input, use \`alert.sendKeys("Text Input Goes Here");\`
4. Return back to the main window: This is typically done automatically when an alert is dismissed or accepted.
Selenium's alert handling capabilities make it easy to automate tests that involve alerts, confirmations, and prompts.
Managing pop-ups in automated tests
Pop-ups, unlike alerts, can be more complex because they often mimic regular browser windows or even include multiple interactive elements. Automating them involves similar steps to handling multiple browser windows:
1. Identify the pop-up: This can be more complex than alerts because pop-ups can contain many elements.
2. Interact with the pop-up: This may involve filling out forms, clicking buttons, or even closing the pop-up window.
3. Close the pop-up and return to the main content.
Effectively managing pop-ups is crucial for ensuring that automated tests are comprehensive and that user interactions with pop-ups are correctly captured and tested.
Dynamic Web Elements in Selenium
Handling dynamic content on web pages
Dynamic content on web pages can be quite a challenge for automation tests. Elements such as menus, lists, and dialogs that load or change based on user actions cannot be handled using standard Selenium Webdriver methods alone.
To effectively interact with these elements, you need to use Selenium’s wait strategies like Explicit Wait, which allows you to pause the script until a certain condition is met.
For instance, you can wait until an element becomes clickable or visible on the page. Using these techniques ensures that your tests are more stable and less likely to fail due to timing issues with dynamic content.
Strategies for dynamic elements in automation
When dealing with dynamic elements, one effective strategy is to use adaptable locators like XPath or CSS selectors that can identify elements based on their relationships to others in the page's HTML structure. For example:
- Using contains, starts-with, or ends-with functions in XPath can help match elements whose attributes change dynamically with each page load.
- CSS selectors can be used to target elements that have a specific class added dynamically.
Combining these advanced locators with Selenium’s waiting mechanisms allows for robust interaction with dynamic web elements, significantly enhancing the reliability of your automation tests.
Advanced Concepts in Selenium Webdriver
TestNG Integration for Selenium
TestNG is a highly organized and controlled testing framework; when integrated with Selenium Webdriver, it organizes the automation framework.
One of the excellent features that TestNG supports for complex test suites includes grouping of tests, parallel execution, and parameterization.
Have easy ways to define test dependencies, even prioritize tests with its annotation-based approach that allows creating detailed reports things very important for a high-quality software delivery process.
Data-driven testing using Selenium
Data-driven testing is referred to when test data is combed out from test scripts; there, different test scenarios can be run by a different set of data.
This can then be easily implemented in Selenium using TestNG where test cases can feed off of multiple data providers for the same test case.
This is particularly useful while running form validation, boundary value testing, and negative testing to ensure the applications behave correctly on a large range of inputs.
Page Object Model (POM) with Selenium
The Page Object Model is a design pattern in Selenium that aims to increase code reusability and LONG test maintenance. In POM, every web page is considered as a class and its different elements are declared as variables on that class.
All the operations that are to be performed on these elements are again modeled as methods of that class. All this abstraction makes test scripts cleaner and more readable.
Implementing the POM can significantly improve the maintainability and readability of your test suite, making it easier to update tests when the UI changes.
Best Practices for Selenium Automation
Writing Efficient and Maintainable Automation Scripts
Creating automation scripts in Selenium Webdriver is not only concerned with getting things done but also their repeatability and maintainability.
The clue to achieving this is to follow some best practices. The Page Object Model must be followed since this design pattern is oriented to the management of web elements on different Web pages and to reduce code duplication.
Keep your code clean and understandable. Using meaningful names for variables, keeping methods focused on one operation. Also, regularly update your scripts in accordance with changes in the web application and the environment.
Effective comments, along with documentation, lend a script maintainability by showing the way around for future updates or to another team member.
Tips for Improving Selenium Test Suite Performance
Optimizing the performance of your Selenium test suite can significantly ripple into the overall efficiency of your test processes. Here are some invaluable tips:
- Opt for selective test execution where possible by using test groups or tags. This way, you only run tests relevant to the changes made in the application.
- Implement parallel test execution to maximize the use of resources and reduce the total time taken for tests to complete. Tools like Selenium Grid can be instrumental in this.
- Minimize the use of explicit waits and rely more on implicit waits to make your tests faster and more reliable.
- Keep your browsers and drivers updated to the latest versions to ensure optimal performance and compatibility.
- Regularly review and refactor your test codes to remove redundancies and outdated methods.
By adopting these strategies, your Selenium tests will not only run faster but also become more robust and easier to manage.
Book a Demo and experience ContextQA testing platform in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
In wrapping up our tutorial, we've seen how Selenium WebDriver, combined with Java, can serve as a powerful toolkit in your automation testing arsenal.
From setting up your environment and understanding basic concepts to interacting with web elements and handling various challenges like waits and iframes, this guide has covered essential steps to help you start or enhance your journey in automation testing.
By following the examples provided, you can extend your testing capabilities and ensure more robust web applications. Moreover, as you practice and integrate these techniques, you'll discover more about Selenium's potential and how it can be tailored to fit specific project needs.
Whether you're a beginner or an experienced tester, the versatility and depth of Selenium WebDriver make it an invaluable resource in the world of software development and quality assurance.
Remember, continuous learning and application are key to mastering Selenium WebDriver. Happy testing!
Also Read - What is Xcode: Features, Installation, Uses, Advantages and Limitations