XPath, short for XML Path Language, is a powerful tool used for navigating through the elements and attributes in an XML document. In the context of web testing and scraping, Selenium utilizes XPath to locate and interact with specific elements on a webpage.
Understanding XPath syntax and its application in Selenium can significantly enhance your ability to automate web browsers for testing or data extraction.
This guide provides an in-depth look at how to effectively implement XPath within the Selenium framework, complete with practical examples to illustrate various techniques.
Utilizing XPath in Selenium allows testers and developers to pinpoint elements with precision, making automated tests more efficient and reliable.
Whether you're new to web testing or looking to refine your automation skills, mastering XPath in Selenium is a valuable skill set that can greatly improve your testing processes.
Understanding XPath in Selenium
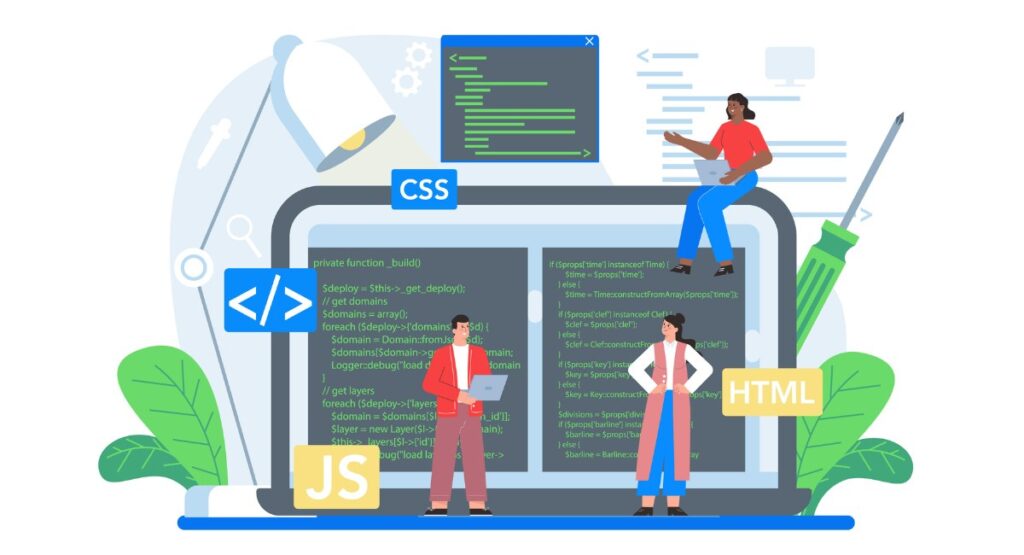
The Significance of XPath in Web Development
XPath, or XML Path Language, serves as a crucial tool in web development for navigating through the XML structure of web documents to locate elements.
Its usage extends beyond XML, as it is equally effective in navigating HTML documents, which form the backbone of the web. With the proliferation of web applications, developers and testers need precise methods to interact with elements within these documents.
XPath enables this precision, allowing for specific elements to be selected, irrespective of their position in the document's hierarchy. This is especially useful in dynamic web pages where elements might not have unique identifiers or stable positions.
Benefits of using XPath in Selenium
Utilizing XPath in Selenium automation testing provides several advantages:
- Flexibility: XPath expressions allow testers to traverse through the XML structure of a web page dynamically. This flexibility helps in locating elements that are difficult to identify with other locator strategies.
- Powerful and Precise: XPath can locate nested elements and elements that share similar attributes with a high level of precision. This is particularly useful in complex web applications with many similar elements.
- Dynamic Targeting: XPath supports functions that can dynamically locate elements based on changing content, attributes, and relationships among other elements. This capability is vital for testing web applications that generate content on the fly.
Basics of XPath
Syntax of XPath
The basic syntax of XPath starts with the double forward slash \`//\`, which selects nodes from the document starting from the current node that match the selection no matter where they are.
After \`//\`, you specify the tag name of the node. For example, \`//div\` selects all \`div\` elements in the document. If you want to specify attributes, you use square brackets. For example, \`//input[@type='text']\` targets all text input elements.
Types of XPath
There are primarily two types of XPath used in Selenium:
- Absolute XPath: This starts from the root node and navigates down to the desired element, specifying every tag in the hierarchy (e.g., \`/html/body/div\`). This type is brittle as any change in the hierarchy requires changing the XPath.
- Relative XPath: More commonly used, starts with the double forward slash \`//\` and allows for the selection of elements relative to anywhere in the document. This type tends to be more flexible and robust against changes in the document's structure.
XPath Axes
XPath axes are methods used to define nodes in a document relative to a current node. Some of the commonly used axes in Selenium are:
- child: Selects all direct children of the current node.
- parent: Selects the parent of the current node.
- ancestor: Selects all ancestors (parent, grandparent, etc.) of the current node.
- sibling: Selects all siblings (shared parent) of the current node.
- preceding: Selects all nodes that come before the current node in the document.
- following: Selects all nodes that come after the current node in the document.
These axes provide powerful ways to navigate around the DOM and locate elements that might otherwise be very difficult to pinpoint using simpler methods.
XPath Locators in Selenium
Different ways to locate elements using XPath
XPath, a powerful tool in Selenium, allows testers to navigate through the structure of a webpage's HTML. XPath locators enable the selection of elements based on their attributes, content, and relationship with other elements.
There are primarily two types of XPath expressions: absolute and relative. Absolute XPath starts from the root node and moves down to the desired element, detailing the entire path.
For example, html/body/div[1]/section/article[1]/ul/li[3]/a. On the other hand, relative XPath, which begins with double slashes (//), can start from anywhere in the document. It is generally preferred because it is shorter and less likely to break with changes in the page structure.
Examples of using XPath locators in Selenium
To demonstrate how XPath can be used in Selenium to locate web elements, consider an HTML page with a list of items. If you want to select the third item in a list with the class 'example', the XPath expression would be:
\`\`\`python
driver.findelementby_xpath("//ul[@class='example']/li[3]")
\`\`\`
This code snippet instructs Selenium to find an unordered list (\`
- \`) with a class attribute of 'example' and then select the third list item (\`
- \`) within it. If you need to locate an element using text, XPath provides the \`text()\` function. For example, to find a link with the text "Click Here":
\`\`\`python
driver.findelementby_xpath("//a[text()='Click Here']")
\`\`\`
Advanced XPath Techniques
Using functions in XPath
XPath functions add flexibility and power to locators. Functions such as \`contains()\`, \`starts-with()\`, and \`last()\` are particularly useful in web testing. For instance, if you want to find a button that contains the word 'Submit', even as part of a larger string like 'Submit Form', you can use:
\`\`\`python
driver.findbyxpath("//button[contains(text(), 'Submit')]")
\`\`\`
This is helpful when dealing with dynamic content where the exact text is unpredictable or when the text includes extra characters due to styling or spacing.
Creating dynamic XPath
Dynamic XPath expressions adapt to changes in the content or structure of web pages, making your tests more robust. For example, if an attribute like an ID changes dynamically, you can use XPath with the \`starts-with()\` or the \`contains()\` function:
\`\`\`python
driver.findelementby_xpath("//input[starts-with(@id, 'user')]")
\`\`\`
This locator will match any input element whose ID starts with 'user', catering to scenarios where the ID might be followed by a numeric or date stamp like 'user123' or 'user20230912'.
Avoid and overcome common XPath pitfalls
One of the key challenges when using XPath is constructing expressions that are not only effective but also efficient and maintainable.
Avoid using absolute XPaths as they are brittle and subject to frequent breakage whenever there's a change in the page layout. Instead, prefer relative XPaths that focus on unique attributes or clear hierarchical paths.
Furthermore, refrain from overly complex XPaths. Layering too many predicates or traversals can make your XPath slow and difficult to understand or debug. Being judicious in the use of wildcards and axes ensures your tests are fast, reliable, and easier to maintain.
Practical Examples of XPath in Selenium
Use case 1: Web scraping with XPath
XPath can be a powerful tool for extracting data from websites during web scraping tasks. For instance, if you need to gather titles from a news website, XPath allows you to selectively navigate through the HTML structure of the webpage. Here’s an example: Suppose we want to scrape all news headlines from a website where each headline is contained within an \`
\` element with a class attribute of 'news-title’. The XPath expression would be: \`//h2[@class='news-title']/text()\`. This XPath query selects the text inside all \`
\` elements with a class of 'news-title'. In your Selenium script, you can iterate through these elements and extract the data as follows:
\`\`\`python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://example-news-website.com")
headlines = driver.findelementsby_xpath("//h2[@class='news-title']/text()")
for headline in headlines:
print(headline.text)
driver.quit()
\`\`\`
Use case 2: Automated testing with XPath
In automated testing, XPath helps ensure that the elements interacted with are the correct ones, especially when IDs and class names are dynamic or similar across multiple elements. For example, to verify a user profile update feature, you might check if the updated name appears in a specific section.
The XPath could be: \`//div[@id='profile']/h1[contains(text(), 'Updated Name')]\`. In a Selenium script, this could be used to navigate to the user's profile after an update and affirm that the name displayed is accurate.
\`\`\`python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://example-social-network.com/user/profile")
nameelement = driver.findelementbyxpath("//div[@id='profile']/h1[contains(text(), 'Updated Name')]")
assert name_element.text == 'Updated Name', "The profile name did not update correctly."
driver.quit()
\`\`\`
Best Practices for Using XPath in Selenium
Tips for writing efficient XPath expressions
Writing efficient XPath expressions can significantly enhance the performance of your scripts. Here are some tips:
- Always use relative paths (\`./\`) instead of absolute paths to make your XPaths shorter and faster.
- Utilize conditions within your XPath expressions efficiently. For example, using \`[contains()]\` or \`[starts-with()]\` functions to target elements with partially matching attributes.
- Avoid using wildcard (\`*\`) excessively, as it can degrade performance by searching through all elements.
Handling dynamic elements with XPath
Dynamic web elements, such as those that change ID or class names on page reload, can be challenging to handle. However, XPath provides functions that can help manage these effectively:
- Use the \`contains()\` function to locate elements by a part of their attributes. For example, \`//*[contains(@id, 'message')]\` can match elements whose \`id\` attribute contains 'message'.
- Leverage sibling and ancestor axes to target elements that are in specific relationships with other elements. For example, finding an input box related directly to a label with the text "Email": \`//label[contains(text(),'Email')]/following-sibling::input\`.
Utilizing these strategies will enhance the accuracy and robustness of your XPath queries in Selenium for both web scraping and automated testing scenarios.
Book a Demo and experience ContextQA testing platform in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
Recap of the importance of XPath in Selenium
XPath provides a powerful way to locate and interact with elements in a web page using Selenium, making it vital for effective web scraping and testing.
Its ability to navigate through the HTML structure of a webpage using various conditions and operators allows for pinpoint accuracy in element selection, which is crucial for dynamic and complex web applications.
Final thoughts and encouragement to practice XPath for web automation.
Mastering XPath within Selenium truly enhances your capabilities in web automation. The versatility and precision offered by XPath make it an indispensable tool in your automation toolkit.
To become proficient, regular practice is key. Experiment with different XPath expressions, understand how slight changes in the path can alter your results, and familiarize yourself with common pitfalls.
The more you practice, the more intuitive finding the right elements on a page will become. Embrace the challenge and always strive to refine your XPath skills!
Also Read - 18 Best Android Emulators For Windows & Mac In 2024