UI testing forms an essential part of the software development cycle to guarantee an app is user-friendly, working according to expectations, and error-free, especially when conducting UI tests in Java.
Among the effective tools to streamline this process is Selenide a very powerful framework designed for automation testing in Java environments.
Based on how easy it is to work with the Selenium API, Selenide enhances its potential and offers expressive and reliable functionality for writing UI tests.
It simplifies the code base because of the automatic browser interactions and rich functionality of methods to interact with web elements.
Besides, setup is minimal and allows developers to pay more attention to the logic of the tests, not to boilerplate code.
This getting started guide will show you the main benefits, setup, and basic usages of Selenide on your way toward more efficient UI testing workflows.
What is Selenide for UI Tests in Java?
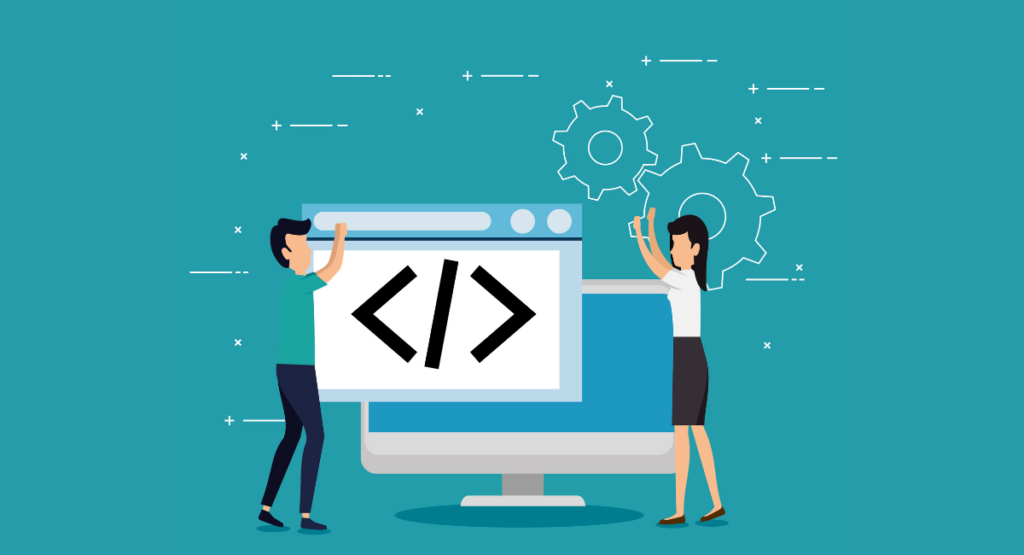
Selenide is an open-source Java library that allows easy building of automated UI tests. Tests are executed by means of the Selenium WebDriver interface, and it wraps them in extended functionality to reduce boilerplate code that should be written to get really robust tests.
Selenide mostly emphasizes simple and fast constructs to enhance the readability of test code and prevent them from becoming a burden.
Features of Selenide
Selenide boasts a variety of features that streamline the process of UI testing in Java:
- Automatic WebDriver Management: Selenide automatically starts and stops browser instances, relieving you of manual WebDriver configuration.
- jQuery-like Selectors: It allows for concise and powerful selectors using methods similar to jQuery, making it easier to locate and interact with elements.
- Built-in Assertions: Selenide includes built-in assertions that help verify conditions within tests, reducing the amount of assertive code you need to write.
- Ajax Support: The framework seamlessly handles modern web technologies like Ajax, ensuring that dynamic content is loaded before actions are taken.
- Screenshots on Failure: Selenide automatically captures screenshots and logs when a test fails, providing immediate feedback for debugging.
Advantages of using Selenide
Opting for Selenide in your Java projects provides numerous advantages:
- Conciseness: The API reduces code complexity and improves readability.
- Less Boilerplate: With the automatic management of WebDriver instances and simpler syntax, less setup and configuration code is required.
- Robustness: Selenide's smart wait mechanisms make tests more reliable by ensuring elements are ready before interactions.
- Easy Integration: It integrates smoothly with popular testing frameworks like JUnit and TestNG.
- Improved Debugging: Embedded reporting tools facilitate easier troubleshooting of failed tests.
Getting Started with Selenide
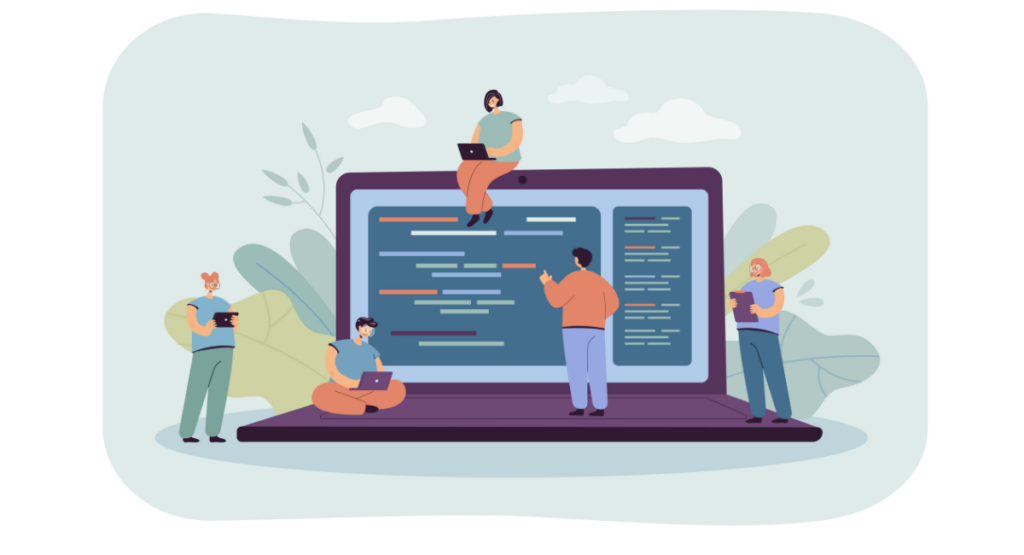
To begin using Selenide, you first need to set up your development environment correctly.
Installation and setup
To install Selenide, you need to include its dependency in your project’s build automation tool. For Maven users, add the following dependency to your \`pom.xml\`:
\`\`\`xml
com.codeborne
selenide
LATEST_VERSION
\`\`\`
For Gradle, include:
\`\`\`gradle
dependencies {
testImplementation 'com.codeborne:selenide:LATEST_VERSION'
}
\`\`\`
Ensure your project is configured to use Java 8 or higher, as this is a requirement for Selenide.
Writing your first UI test with Selenide
Creating your first UI test with Selenide involves only a few steps:
- Creating a Test Class: Create a test class with the aid of a testing framework, for example, JUnit.
- Opening a Web Page: Apply Selenide's \`open(url)\` method for opening the intended page.
- Performing Actions and Assertions: Interact with the elements of the page using methods provided by Selenide, validate the expected results.
Example:
\`\`\`java
import static com.codeborne.selenide.Selenide.*;
import static com.codeborne.selenide.Condition.*;
public class GoogleTest {
@Test
public void userCanSearch() {
open("https://www.google.com");
$("input[name='q']").setValue("Selenide").pressEnter();
$("h3").shouldHave(text("selenide.org"));
}
}
\`\`\`
Understanding SReselenide's syntax
Selenide's syntax is intuitive, leveraging human-readable commands to interact with web elements:
- Open a URL with \`open()\`: Directs the browser to a specific location.
- Finding elements with \`$()\`: Similar to jQuery, it allows for selecting elements based on CSS or XPath queries.
- Interactions like \`click()\`, \`setValue()\`: Perform user interactions on the web elements.
- Assertions such as \`shouldHave()\`: Check conditions like visibility, text content, and more to assert expected states of elements.
By leveraging these functionalities, Selenide allows developers to write concise, maintainable, and robust UI tests.
Advanced Features of Selinide
Modern web applications often feature complex, dynamic user interfaces that can make automated testing a challenge. Selenide, however, offers several advanced features specifically designed to handle these elements efficiently.
Handling dynamic elements
Dynamic elements are those that may not always be present on the webpage or whose properties might change based on certain actions.
Selenide simplifies interactions with such elements through its robust API. It automatically waits for elements to appear or become clickable before performing any actions, thereby reducing the chances of tests failing due to timing issues.
Additionally, Selenide's \`$\`, \`$$\`, and \`shouldBe\` methods allow you to handle elements that appear dynamically with ease, ensuring that your tests are both robust and reliable.
Dealing with pop-ups and alerts
Pop-ups and alerts can disrupt the flow of a test and potentially make it fail if not handled properly. Selenide provides easy ways to handle such interrupts.
For instance, you could use \`confirm()\`, \`alert()\`, and \`prompt()\` to deal with alert boxes and confirm dialogs, closing or accepting as appropriate for your test.
Such functionality is useful in cases when you need to check if some alert has appeared under certain conditions, or just to continue the flow of the test after treatment of an unexpected pop-up.
Using Selenide with test frameworks like JUnit or TestNG
Selenide seamlessly integrates with popular Java test frameworks such as JUnit and TestNG. This integration enhances test management and allows you to combinethe descriptive capabilities of these frameworks with the concise syntax of Selenide.
For example, using Selenide with JUnit allows you to take advantage of annotations like \`@Before\`, \`@After\`, \`@Test\`, etc., to structure your tests clearly and maintainably.
This integration not only streameer controls but also improves the readability and organization of your test suites.
Best Practices for Effective UI Testing with Selenide
Effective UI testing requires a balance between speed, reliability, and maintainability. Selenide, with its concise API, helps achieve this balance, especially when implemented with some best practice strategies.
Locator strategies for robust tests
Choosing the right locators is crucial for creating stable and reliable UI tests. In Selenide, it is advisable to use ID, class, or CSS selectors, which are less likely to change than XPath locators.
Employing such strategies reduces the likelihood of tests breaking with changes in the UI. Additionally, Selenide allows for the creation of custom selectors when predefined ones don't meet your requirements, offering further flexibility and robustness in test design.
Writing maintainable and scalable UI test suites
To ensure that your Selenide tests are both maintainable and scalable, organize them in a logical, modular fashion. Use Page Object Models to encapsulate the structure and behavior of pages within your application, and employ methods that are reusable across different tests.
Furthermore, keeping your tests clear and focused on one functionality per test promotes easier maintenance and scaling as your application evolves.
Integrating Selenide with Continuous Integration tools
Integrating Selenide tests into a Continuous Integration (CI) pipeline, such as Jenkins, Bamboo, or CircleCI, can significantly enhance the quality assurance process.
This integration enables automated triggers for your tests, ensuring they are run at pivotal points, such as before a deployment or after a significant merge in source control.
Using Selenide with CI tools helps in identifying issues early and streamlines the testing process, facilitating a more agile development environment.
Book a Demo and experience ContextQA testing tool in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
Selenide is an innovative solution for UI testing in Java that simplifies the creation and maintenance of tests.
Its straightforward syntax, built-in functionalities, and automatic handling of common issues like timeouts and element visibility significantly reduce the complexity and amount of code you need to write.
By integrating Selenide into your development process, you can enhance the reliability of your tests, increase test coverage, and speed up the testing cycle.
This can lead to early detection of UI bugs and ultimately contribute to delivering a higher quality product. Whether you're a novice in automation testing or an experienced tester looking for a more efficient tool, Selenide offers a compelling option that aligns with modern software development practices.
Also Read - Advanced Automated Visual Testing With Selenium: Best Practices, Techniques!
We make it easy to get started with the ContextQA tool: Start Free Trial.