In the world of programming, particularly in Python, managing errors efficiently is crucial for building robust applications. One powerful tool provided by Python for such a task is the assert statement.
Although simple, the assert statement is a vital part of Python's error handling and debugging arsenal. Primarily used during development stages, it helps programmers check conditions that must always be true and quickly pinpoint bugs.
In this blog, we will explore what the assert statement is, understand its syntax, and learn how to effectively apply it in Python programming for optimal error handling and debugging.
Understanding the assert statement in Python
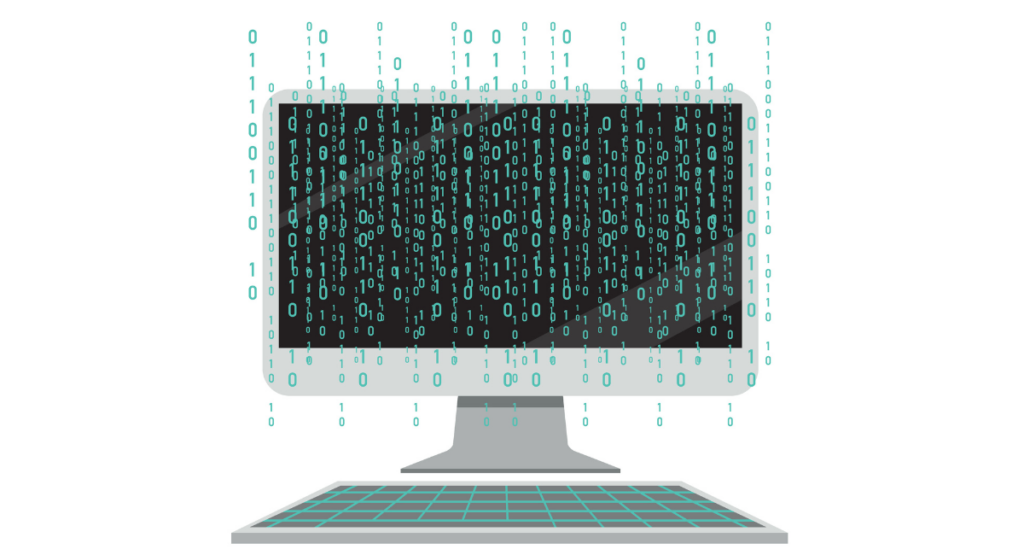
What is the assert statement?
The Python assert statement is a debugging aid that allows one to check conditions and see if they are true or not. If the condition is true, then the program continues without any issues at all; otherwise, it stops if it's false and throws an AssertionError.
Of course, this feature is mainly used only during the development phase so that bugs may be caught early in the development cycle.
Assertions are especially useful as sanity checks, which are compiled in for debugging but discounted for the final release in production.
Why use assert in Python?
Using assert in Python helps programmers catch issues in an application at the point of their occurrence and provides a clear indication of the failure. Here are a few reasons why assert is valuable:
- Early detection of bugs: Since asserts stop the program immediately when a problem is detected, it’s easier to locate the source of the issue.
- Documentation: Assert statements also serve as a form of live documentation. When reading code, seeing an assert statement explains what conditions are expected, increasing code readability and maintainability.
- Safeguarding: By ensuring that critical assumptions are true, assert statements safeguard the functioning of the program under expected conditions.
Syntax of the assert statement
Basic syntax of assert in Python
The basic syntax of an assert statement in Python is straightforward. It consists of the keyword 'assert' followed by a Boolean expression and optionally, a comma-separated error message for when the expression yields 'False’. The structure is:
\`\`\`
assert condition, errormessageoptional
\`\`\`
For example:
\`\`\`
assert x > 0, "x should be greater than 0"
\`\`\`
Here, Python checks if x is greater than zero and if not, raises an AssertionError displaying the message.
Using assert with conditionals
Assert statements can be strategically placed after conditional statements to check the validity of outcomes and state conditions clearly. For instance:
\`\`\`
x = 10
assert x > 0, "x is not a positive number"
if x > 0:
print("Processing")
assert x < 50, "x is too large to process efficiently"
# Proceed with code, knowing x is within the acceptable range.
\`\`\`
In this example, the first assert checks if x is positive, then, if positive, it moves onto a block that requires x to be less than 50 for efficient processing.
This use of asserts after conditional checks ensures that the program’s flow matches your expectations and any deviations are caught early on.
How to use assert for efficient error handling
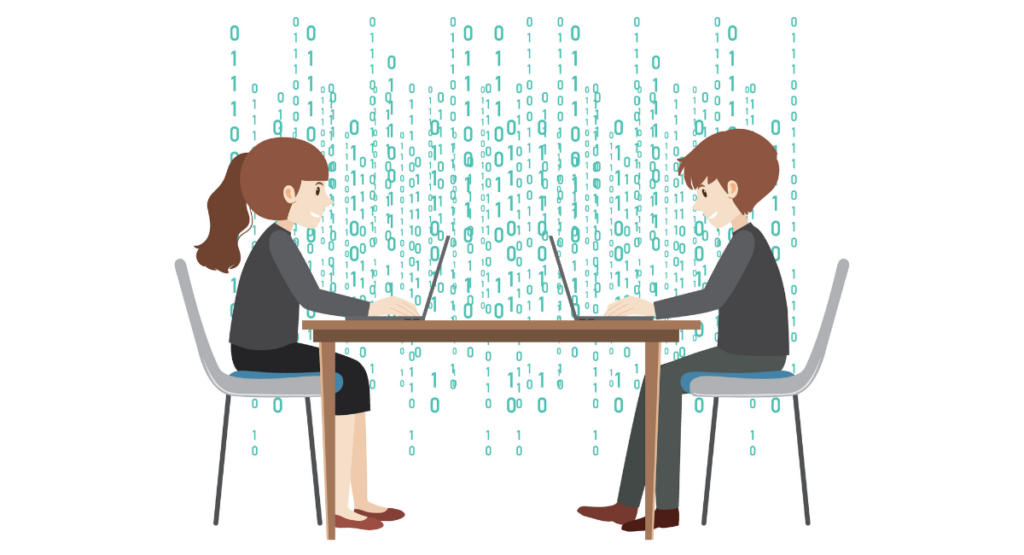
The Python assert statement enormously enhances the reliability and consistency of your code while it is under development.
This makes it easier on you to know where to look while searching for a bug and forces some conditions to occur in your code, which otherwise might be handled by many if statements, making source code management and debugging easy.
Assert for input validation
Input validation is critical in ensuring that functions or methods are receiving appropriate data. By using assert statements, you can check for valid inputs before processing them.
This preemptive check helps prevent bugs and errors later in your code's execution. For example, if a function requires a positive integer to operate correctly, you can assert that the input is an integer and greater than zero.
If the condition fails, the program will raise an AssertionError with an optional message you can specify.
Assert for debugging code
During the debugging process, asserts can serve as internal self-checks for your code. They ensure that specific conditions are met before the code proceeds further, which can help isolate problematic sections when something goes wrong.
This is particularly useful in large, complex systems where the cause of errors might not be immediately apparent.
Best practices for using assert in Python
When utilizing assert statements, there are several best practices to follow:
- Use asserts to check for conditions that should never happen but are not intended to handle programmable errors.
- Avoid using asserts for data validation in production code; exceptions are more suitable for this purpose.
- Keep the error messages informative to clarify why the assertion failed.
Examples of assert in Python
Example 1: Simple assert statement
A straightforward example of using assert:
\`\`\`python
def calculate_average(numbers):
assert len(numbers) > 0, "The list of numbers should not be empty"
return sum(numbers) / len(numbers)
\`\`\`
This ensures the function does not proceed with an empty list, which would cause a division by zero error.
Example 2: Assert with conditionals
You can also couple assert statements with more complex conditional logic:
\`\`\`python
def process_order(order):
assert order.status == 'paid' and order.items > 0, "Order must be paid and have more than zero items"
print("Processing order...")
\`\`\`
This checks multiple conditions at once, making sure the order is paid and contains items.
Example 3: Assert for debugging a function
Asserts can be incredibly useful for debugging functions by confirming intermediate results:
\`\`\`python
def addpositivenumbers(x, y):
assert x > 0 and y > 0, "Both numbers must be positive"
result = x + y
assert result > x and result > y, "Result should be larger than both numbers"
return result
\`\`\`
This checks not only the input but also the logic of the function itself.
Example 4: Implementing assert in a real-life scenario
Consider an application managing user registration:
\`\`\`python
def register_user(username, age):
assert isinstance(username, str), "Username must be a string"
assert isinstance(age, int) and age >= 18, "User must be at least 18 years old"
print(f"Registering user {username}!")
\`\`\`
This implementation ensures that the data types and age conditions are met before a user can be registered.
Common mistakes and pitfalls when using assert
Misusing the assert statement
Assert statements should be utilized to check for conditions that must always be true unless there's a bug in the program. However, one common misuse is relying on assert statements to handle business logic or user input validation.
This approach is flawed because asserts can be disabled in optimized modes running Python with the \`-O\` or \`-OO\` flags, which strips out these statements.
Consequently, critical validations or logic checks might not be performed, leading to unexpected behaviors or security vulnerabilities. Moreover, using asserts for validating external conditions like file existence or user inputs makes the code less robust and secure.
Handling assert errors effectively
To effectively handle errors highlighted by assert statements, it's essential to understand that these are indicative of underlying bugs in the code rather than expected runtime errors.
When an \`AssertionError\` is raised, it should prompt an immediate investigation into why the presumed impossible condition occurred. Here are some strategies for handling such errors:
- Immediate Logging: Capture comprehensive log details at the point the assert fires. This will help in diagnosing what went wrong.
- Informative Messages: Always provide a clear, informative message with each assert statement. This message should explain what was expected and why the assertion failed, aiding in quicker debugging.
- Testing and Validation: Regularly test parts of your code where asserts are used. This practice ensures that changes in code or environment do not invalidate the assumptions made in these assertions.
By being mindful of these considerations, developers can leverage Python's assert mechanism as a powerful tool for maintaining code correctness and robustness.
Book a Demo and experience ContextQA testing tool in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
In summary, the statement assert is useful in checking that some condition in your program has been satisfied at runtime.
It strengthens your debugging by catching errors at a very pristine stage, hence making your code more robust and maintainable.
Remember, assert should be best used during the development phase for internal testing and should not replace proper error handling in production code.
Properly used, assert can greatly reduce the effort of developing and maintaining complex Python applications. Use it wisely to sharpen your code and debug it with fewer hassles.
Also Read - Safari Browser Testing on Real Devices, no VMs
We make it easy to get started with the ContextQA tool: Start Free Trial.