Unit testing is one of the most important parts of software development since it ensures that every unit of the software performs as intended.
Among the famous unit test frameworks in Java, JUnit makes testing easier, more practical, and efficient. Good test cases written in JUnit will let one verify the correctness of your code, catch bugs at the very beginning, and integrate more smoothly.
This blog will help you get started on how to write test cases in JUnit to increase the reliability and maintainability of your Java applications.
Understanding JUnit Test Cases
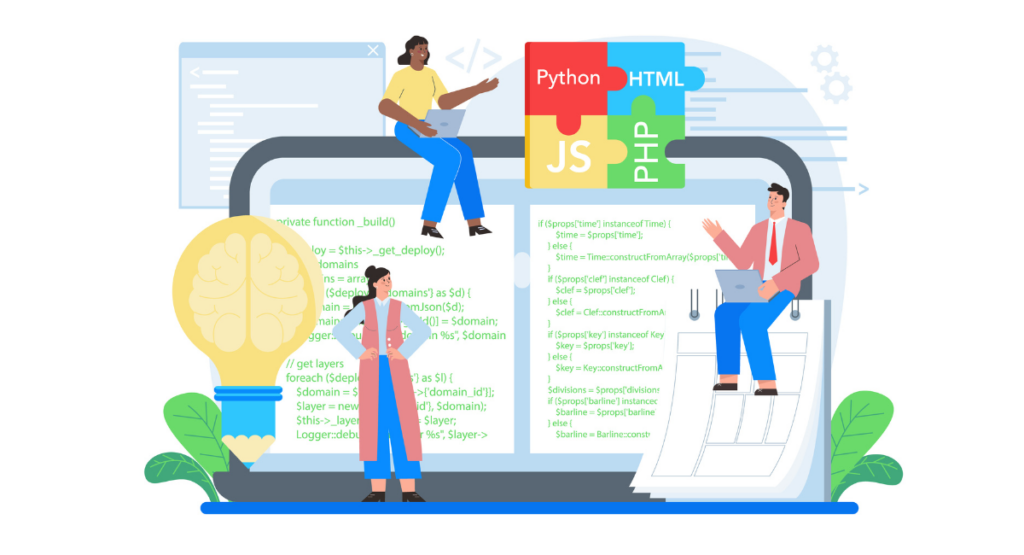
What are JUnit test cases?
A JUnit test case is a small Java code written to test another lengthy Java code. It is used to check that smaller parts or units of an application work as expected.
Typical test cases are organized in such a way that they usually check one given aspect of a method against certain conditions using assertions for the analysis of expected outcomes.
Running independently means JUnit is going to generate a report on success or failure of tests, which then could be used to enhance the quality of software.
Importance of writing JUnit test cases
Writing JUnit test cases is very important in the software development process, as this becomes a means of ensuring the reliability and maintainability of the code base over time. Effective testing using JUnit facilitates the developer to:
- Early detection and fixing of bugs in the development cycle, hence saving development time and cost.
- Quality in relation to the code, ensuring that newly added or modified functionality will not break already working features of the system.
- Easy integration, which checks that all units work together properly.
- Facilitate code changes and refactoring since tests can quickly verify the behavior of modified code.
- Enhance understanding of the code as test cases provide a form of documentation of what the code is supposed to do.
Setting Up JUnit
Installing JUnit in your Java project
First of all, to begin writing JUnit tests, you need to add JUnit into your Java project. Most modern IDEs, such as Eclipse, IDEA, and VS Code, integrate with it pretty straightforwardly.
You can also add it by hand after downloading the JUnit jar file from the official website. You can even do this with the help of build tools like Maven or Gradle just add the JUnit dependency in your \`pom.xml\` or \`build.gradle\` file.
Creating a test class for JUnit test cases
A test class in JUnit is simply a Java class annotated with \`@Test\`. To create one:
- Create a new Java class in your project.
- Add the \`@Test\` import declaration from the JUnit library.
- Define methods within the class that will contain your test cases. Each method tagged with \`@Test\` will be treated as a separate test case by JUnit.
For instance, if you are testing a class named \`Calculator\`, your test class might be named \`CalculatorTests\`.
Using annotations in JUnit
JUnit utilizes annotations to simplify the writing and understanding of test cases. Key annotations include:
- \`@Test\`: Identifies a method as a test method.
- \`@Before\`: Runs before each test; used for setting up test environments.
- \`@After\`: Runs after each test; used for cleanup activities.
- \`@BeforeClass\`: Runs once before any of the test methods in the class; useful for resource-intensive activities like opening database connections.
- \`@AfterTeam\`: Executes once after all tests in the class have run, suitable for cleaning up resources like closing database connections.
- \`@Ignore\`: Marks that the test method should not be executed.
These annotations help structure your test executions and management, making your testing process more efficient and coherent.
Writing Your First JUnit Test Case
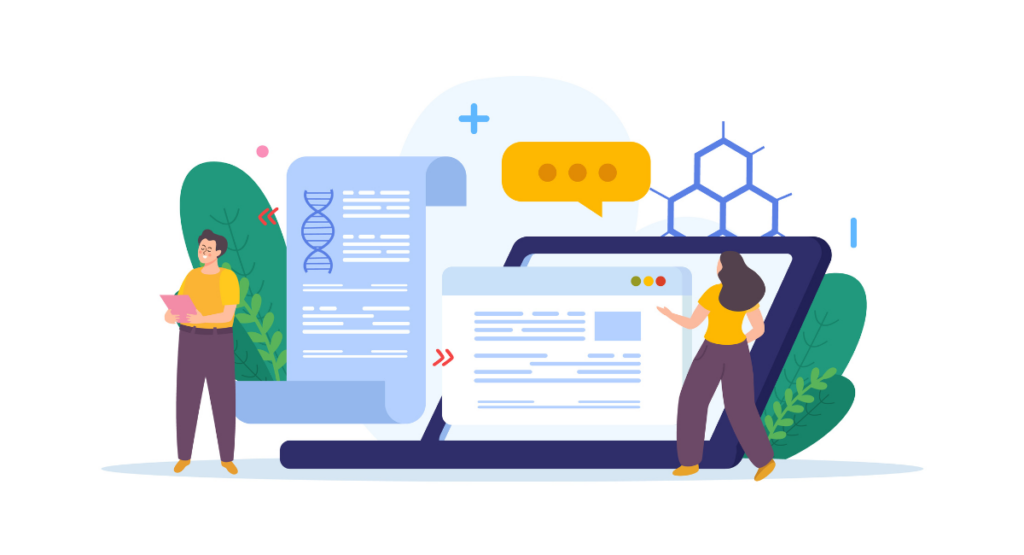
Anatomy of a JUnit test case
A JUnit test case is defined within a Java class where each test method is annotated with @Test.
The basic structure includes setting up data or configurations in a method annotated with @Before, the actual test methods, and any cleanup required in a method annotated with @After.
Each test method should ideally test a single functionality. Here’s a simple example:
\`\`\`java
import static org.junit.Assert.*;
import org.junit.*;
public class ExampleTests {
private ArrayList testList;
@Before
public void setUp() {
testList = new ArrayList();
}
@Test
public void testIsEmpty() {
assertTrue("The list should be empty", testList.isEmpty());
}
@After
public void tearDown() {
testList.clear();
}
}
\`\`\`
Writing assertions in JUnit
Assertions in JUnit are crucial for verifying that the code works as expected. The most common assertions include \`assertEquals\`, \`assertTrue\`, \`assertFalse\`, and \`assertNotNull\`.
These assertions test the conditions exactly as they sound; for instance, \`assertTrue\` passes if the condition is true, and \`assertEquals\` passes if the expected and actual values are equal.
Running your JUnit test cases
JUnit test cases can be run directly within an IDE with built-in JUnit support like Eclipse, IntelliJ IDEA, or NetBeans.
They can also be run from the command line using Maven or Gradle. Typically, all you need to do is trigger the test phase:
\`\`\`bash
mvn test
\`\`\`
This command runs all tests in your Maven project and reports the results.
Best Practices for Writing JUnit Test Cases
Keeping test cases independent and isolated
Each test case should function independently of others. Avoid any dependencies between tests, as this ensures that the failure of one test does not cascade to others.
This practice helps in identifying problems in the codebase quickly and ensures that tests are reliable and easy to maintain.
Using parameterized tests for multiple inputs
JUnit provides support for writing parameterized tests using the \`@Parameterized\` annotation. This allows you to run the same test over a variety of data sets.
It helps in covering multiple scenarios and reduces code duplication. Here’s how you can implement it:
\`\`\`java
@RunWith(Parameterized.class)
public class NumberTest {
private int number;
private boolean expectedResult;
public NumberTest(int number, boolean expectedResult) {
this.number = number;
this.expectedResult = expectedResult;
}
@Parameterized.Parameters
public static Collection data() {
return Arrays.asList(new Object[][] {{1, true}, {2, false}});
}
@Test
public void testNumber() {
assertEquals(expectedResult, NumberChecker.isNumberValid(number));
}
}
\`\`\`
Handling exceptions in JUnit test cases
To test exceptions, you can use the \`expected\` attribute of the \`@Test\` annotation to declare an expected exception or use the \`assertThrows\` method from JUnit 4 onwards to handle and assert exceptions more flexibly.
Mocking objects for unit testing
In unit testing, mocking is essential for isolating the unit of work to determine its correctness without relying on dependencies. JUnit works well with mocking frameworks like Mockito.
Using these, you can simulate the behavior of complex real objects and specify the outputs they should return when certain methods are invoked.
This approach is particularly useful in dealing with external systems or services.
Advanced Techniques in JUnit
Testing JUnit with different frameworks
Combinability of JUnit with other testing frameworks creates ease in writing and running tests, hence improving the development process.
For instance, integration of JUnit with Mockito allows mocking dependencies and improves isolation of units under test, making the conducting of unit tests for services with strong dependencies on external elements very practical.
Another framework that has attracted the eyes of developers is PowerMock. It extends Mockito and helps one to test private methods, static methods, and constructors.
Anyway, when you are testing with two stand-alone frameworks jointly, beware that tests may become complicated and hard to manage when testing for one single functionality.
Integrating JUnit with continuous integration tools
It integrates well with other CI tools like Jenkins, CircleCI, or Travis CI, further empowering any testing strategy.
Such tools will execute all your test suits after every change in your codebase, hence detecting the problems at the earliest time possible and quickly resolving them.
With regards to integrations, be sure that your JUnit tests are returning results in an easily interpretable way by the CI tool, usually through a plugin or intrinsic support.
Automation of your JUnit tests in a CI pipeline is a significant point toward the assurance of code quality and overall feedback loop speed, which are highly desired in agile development practices.
Organizing test cases effectively
Organizing your test cases effectively can greatly enhance their clarity and ease of maintenance. Group related tests within the same test classes or packages based on the functionality they cover.
Utilize descriptive names for your test methods to clarify their purpose without needing to delve into the implementation details.
For larger projects, consider categorizing tests into different levels, such as unit tests, integration tests, and system tests, to simplify navigation and execution.
Writing readable and maintainable test case
Relevant will be writing readable test cases that are maintainable. For the long-term success of the project, always follow a consistent style and naming convention in your test codebase.
Apply comments in such a manner that they will serve the purpose of explaining why a particular test is necessary, or why it is setup in certain way if not immediately obvious.
The test purposes and outcomes could also be understood by clearly employing assertive statements and meaningful assertion messages.
Finally, it is highly recommended to keep your test code as simple and focused as possible; complex tests will tend to be brittle and hard to maintain in the long term.
Book a Demo and experience ContextQA testing platform in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
JUnit testing is a very powerful framework important for the reliability and functionality assurance of Java applications.
By applying these principles from the guide, developers will have effective and thorough JUnit test cases, protecting their software from failures and guaranteeing a good quality end product.
Remember that success in unit testing is built on writing concise tests that are readable and making them part of your software development cycle. Keep improving your skills in testing to get more robust and less error-prone applications.
Also Read - What is IP Whitelisting?