Random string generation is a common requirement in software development, particularly in areas like security for generating passwords, testing databases, and creating unique identifiers. Python, with its extensive libraries and simple syntax, is an excellent tool for this task.
Utilizing Python for string generation not only enhances efficiency but also ensures robustness in applications. In this blog, we will explore various methods to generate random strings in Python, catering to both beginner and advanced programmers.
Whether you need a simple random string for a CAPTCHA or a complex string for cryptographic purposes, Python offers diverse solutions to suit any need.
Understanding Random String Generation in Python
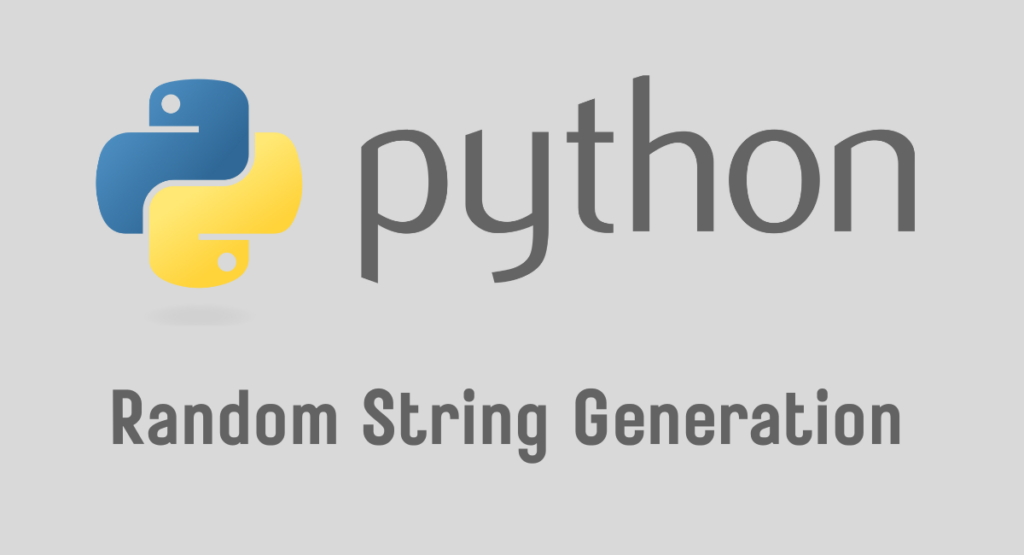
Random string generation is a common requirement in software development for tasks like generating passwords, unique identifiers, and secure tokens.
Python, with its vast array of built-in functions and external libraries, simplifies the process of generating random strings tailored to specific needs. The strings can include a variety of characters such as uppercase, lowercase, digits, and special symbols.
The randomness in Python is achieved using algorithms that generate pseudorandom numbers, which are adequate for most applications but not suitable for cryptographic purposes.
For cryptographic security, Python offers facilities in its standard libraries that ensure the generation of securely random strings.
Using Python Libraries for Random String Generation
There are several methods to generate random strings in Python, each suited to different requirements and scenarios:
Using the \`random\` Module
The \`random\` module in Python can be utilized to generate random strings. Here’s a basic approach:
- Import the \`random\` module.
- Define a string of possible characters.
- Use a loop to select a character at random from the string for as many times as desired to create a string of a specific length.
\`\`\`python
import random
import string
def generaterandomstring(length):
letters = string.ascii_letters + string.digits
result_str = ''.join(random.choice(letters) for i in range(length))
return result_str
\`\`\`
Using the \`secrets\` Module
For generating a random string that requires a higher level of security, such as for passwords or cryptographic keys, Python’s \`secrets\` module is preferable. This module is designed to generate random numbers that are cryptographically strong.
\`\`\`python
import secrets
import string
def securerandomstring(length):
securechars = string.asciiletters + string.digits + string.punctuation
securestr = ''.join(secrets.choice(securechars) for i in range(length))
return secure_str
\`\`\`
By choosing the right module and method, developers can efficiently generate random strings that meet the security and functionality requirements of their applications.
Whether it's for non-critical applications using the \`random\` module or for sensitive ones that opt for the higher security of the \`secrets\` module, Python provides robust options for random string generation.
Generating Random Strings with Python
Random string generation is a common task in software development, especially in situations where unique identifiers or tokens are required. Python, with its extensive standard library and third-party packages, offers several ways to generate random strings effectively.
Using the \`random\` Module
The \`random\` module in Python provides functions that help in generating random data. To create random strings, you can combine these functions with Python's string manipulation features. Here’s a simple way to generate a random string using the \`random\` module:
\`\`\`python
import random
import string
def generaterandomstring(length):
letters = string.ascii_letters + string.digits
return ''.join(random.choice(letters) for i in range(length))
\`\`\`
This function selects random characters from a pool of alphanumeric characters (both uppercase and lowercase) and digits, creating a string of a specified length.
Using the \`secrets\` Module
For more security-sensitive applications, where the randomness needs to be less predictable, the \`secrets\` module is preferable. This module is designed for generating cryptographically secure random numbers and strings, suitable for passwords, account authentication, security tokens, and related use cases.
Example of generating a secure random string:
\`\`\`python
import secrets
import string
def securerandomstring(length):
securestr = ''.join(secrets.choice(string.asciiletters + string.digits) for i in range(length))
return secure_str
\`\`\`
This method ensures that the generated strings are unpredictable, reducing the risk of guessability.
Customizing Random String Generation in Python
Customizing the generation of random strings allows developers to tailor the output to specific needs, such as including special characters or controlling the probability of certain characters appearing.
Including Special Characters
In some applications, you might need to include special characters in your random strings (e.g., for generating more complex passwords). You can easily customize the character set from which the random characters are drawn:
\`\`\`python
import random
def customrandomstring(length, includespecialchars=False):
charset = string.asciiletters + string.digits
if includespecialchars:
char_set += string.punctuation
return ''.join(random.choice(char_set) for i in range(length))
\`\`\`
With the \`includespecialchars\` option, this function can generate strings that are even more secure by incorporating symbols and punctuations.
Adjusting Character Frequency
To control the frequency of certain characters in the generated string, you can modify the character pool by repeating characters to increase their probability of selection. This approach is useful when certain characters need to be emphasized or discouraged:
\`\`\`python
def frequencyadjustedrandom_string(length):
charset = string.asciiletters + string.digits + ('' * 10) # Increasing the frequency of ''
return ''.join(random.choice(char_set) for i in range(length))
\`\`\`
By increasing the count of specific characters in the \`char_set\`, you can skew the randomness in favor of these characters. This method offers a simple way to customize the behavior of your string generation based on the requirements of your application or project.
Advanced Techniques for Random String Generation in Python
Generating random strings in Python can be tailored for more complex requirements beyond basic alphanumeric strings. For situations requiring unique identifiers or tokens, more sophisticated methods might be necessary.
Using UUID for Unique Identifiers
The Python \`uuid\` module provides a robust method for generating universally unique identifiers (UUIDs). Here’s how to generate a UUID as a string:
\`\`\`python
import uuid
unique_string = str(uuid.uuid4())
print(unique_string)
\`\`\`
This method ensures that each generated string is unique across every computer in the world, which is perfect for applications requiring a high level of uniqueness, such as database keys.
Combining Characters and Special Symbols
Sometimes, it’s beneficial to include special symbols along with alphanumeric characters to increase the complexity of the generated string. You can easily integrate symbols by expanding the character set:
\`\`\`python
import random
import string
length = 10
chars = string.ascii_letters + string.digits + string.punctuation
randomstring = ''.join(random.choice(chars) for in range(length))
print(random_string)
\`\`\`
This can be particularly useful for generating passwords or codes that require additional security.
Practical Examples of Random String Generation in Python
Let’s apply what we’ve learned with some practical examples. These instances demonstrate fitting use cases of random string generation.
Creating Random Passwords
To create a random password, blend uppercase and lowercase letters, numbers, and special symbols:
\`\`\`python
import random
import string
def generate_password(length):
chars = string.ascii_upperourscape + string.digits + string.punctuation
return ''.join(random.choice(chars) for _ in range(length))
password = generate_password(12)
print(password)
\`\`\`
This method is straightforward but very effective for generating secure passwords.
Random URL Slug Generation
For web developers, generating slugs for URLs can be done randomly which ensures uniqueness:
\`\`\`python
import random
import string
def generate_slug(length):
chars = string.ascii_lowercase + string.digits
return ''.join(random.choice(chars) for _ in range(length))
slug = generate_slug(8)
print(slug)
\`\`\`
This example showcases generating a random slug that could be used for any URL needing a unique address in a web application.
Best Practices and Tips for Efficient Random String Generation
When generating random strings in Python, it’s important to focus on efficiency and security, particularly in application scenarios that involve sensitive data or require high performance. Here are some best practices and tips to ensure that your string generation is both efficient and effective:
- Keep It Simple: Utilize built-in Python libraries like \`random\` or \`string\` whenever possible. These libraries are optimized for performance and are thoroughly tested for reliability.
- Use the Right Tools: For more security-sensitive applications, consider using the \`secrets\` module, which is designed for generating cryptographically strong random numbers and strings.
- Pre-define Length: Where possible, pre-define the length of the strings to avoid unnecessary computation and memory usage. This also helps in consistent data formatting across your application.
- Avoid Bias in Characters: When using custom functions for random string generation, ensure that each character has an equal probability of being selected.
This prevents certain characters from appearing more frequently than others, which can be crucial for maintaining data integrity and security.
- Test Extensively: Always test your random string generation code under various scenarios to ensure its robustness. This includes testing with different lengths, character sets, and in different operating environments.
By following these guidelines, you can maximize the performance and security of your random string generation methods in Python, making your programming projects more reliable and effective.
Book a Demo and experience ContextQA testing platform in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
In conclusion, Python provides several efficient methods for generating random strings, making it an invaluable tool for developers needing unique identifiers, passwords, or testing data.
From utilizing the built-in \`random\` module to exploring external libraries like \`secrets\` for security-focused applications, Python offers versatility and power.
Mastering these techniques can greatly enhance your programming projects, ensuring robust and effective code implementation. Remember to choose the method that best aligns with your project's security requirements and performance needs.
Also Read - 80+ Best Chrome Extension Ideas For 2024: You Must Know