Struggling with an "Uncaught TypeError: Cannot Read Properties of Undefined" error in JavaScript can be exasperating for developers of all levels. This issue typically arises when attempting to access a property or invoke a method on an undefined element in your code.
Enhancing your debugging abilities and saving time is possible by grasping the usual triggers and remedies for this error. This article examines the reasons behind this error and offers effective methods to resolve it.
Understanding Uncaught TypeError: Cannot Read Properties of Undefined
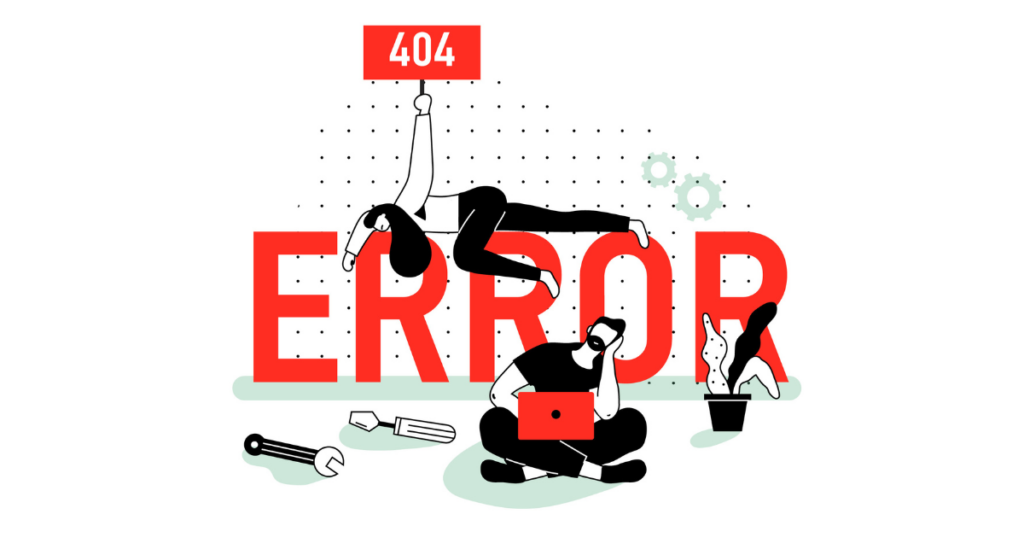
Definition and common scenarios
A JavaScript error of "Uncaught TypeError: Cannot read properties of undefined" occurs when attempting to access a property or method of a variable that is undefined.
This frequently occurs in many situations: working with an object that has not been set up, reaching beyond the limits of an array, or using functions that do not explicitly give back a value.
Basically, this error means that in the code, an action is being tried on a variable that is either declared but not defined or not declared at all.
Impact on JavaScript code
This type of error can severely impact the performance and functionality of JavaScript applications. It causes the execution of the current function to stop, and, depending on the JavaScript environment, may stop the execution of all subsequent scripts.
This leads to functionality breakdowns, user experience issues, and can potentially expose your application to security vulnerabilities due to improper data handling.
Causes of Uncaught TypeError
Accessing undefined variables
One of the most straightforward causes of this TypeError is accessing undefined variables. This typically happens when variables are used in the code without being correctly declared or initialized. For instance:
- Using a variable before it is declared.
- Typographical errors in variable names.
- Logical errors, such as out-of-scope variable access.
Inconsistent data types
Attempting to access properties on data types that are not objects or arrays can also lead to this error. JavaScript is a loosely-typed language, meaning data types are automatically converted during the execution of the code.
If an operation expects an object, but receives a different data type like null or undefined, it will result in this TypeError.
Undefined object properties
Accessing properties or methods of an object that does not exist is another common cause. This can occur in several ways:
- When an object is expected from a function or an API, but the function returns undefined or null.
- Property names that don’t exist on the expected object.
- Errors in asynchronous code execution where the object is not yet defined.
Understanding these typical causes and their implications can significantly help in debugging and preventing the "Uncaught TypeError: Cannot read properties of undefined" error in JavaScript applications.
Troubleshooting Uncaught TypeError
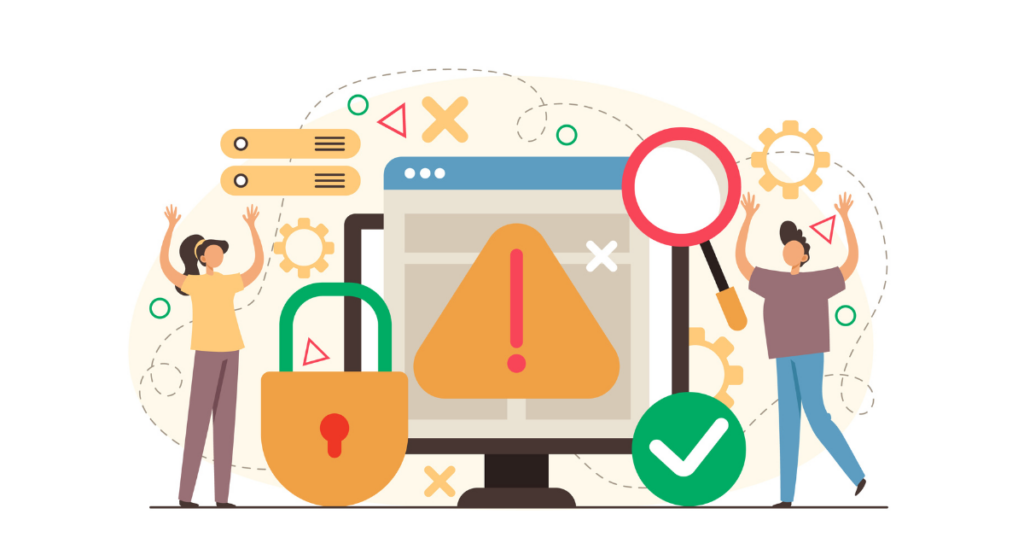
Debugging techniques
Troubleshooting an Uncaught TypeError begins with identifying the part of the code where the undefined variable is referenced. Carefully analyze the stack trace provided in the error message, which gives clues about where and when the undefined reference occurs.
Follow the flow of function calls leading up to the error, and check if any object or variable is not properly initialized before its properties are accessed. Keeping your functions small and responsibilities clear can simplify this process significantly.
Using console.log() for variable tracking
\`console.log()\` is a powerful tool for debugging. Insert \`console.log()\` statements before the error occurs to print out the values of variables and objects at various stages of execution.
This method helps in understanding what data each variable holds at different points in time. It’s particularly useful for tracking down where a variable might be getting set to undefined.
Ensure to remove or comment out these debugging lines after resolving the issues to keep the console clean and maintain the performance of your application.
Utilizing browser developer tools
Most modern browsers come equipped with developer tools that provide a debugger and other utilities which can pause the JavaScript execution and inspect the values held by variables at any point in time.
Set breakpoints near where the error occurs and step through the code line-by-line. Watch the expressions and evaluate how each statement affects the program's state.
These tools can also highlight logical errors that lead to a variable becoming undefined unexpectedly.
Preventing Uncaught TypeError in JavaScript
Best practices for variable declarations
A fundamental way to prevent these types of errors is to adhere strictly to best practices for declaring variables. Always declare variables with \`let\` or \`const\` before using them, which ensures they are scoped correctly.
Avoid using \`var\` if possible, as it can lead to unexpected global variables due to its function-scoped nature. Use \`const\` by default to protect variables from being reassigned accidentally, which can lead to unexpected behavior or accessing undefined properties.
Null and undefined checks
Before accessing properties of an object, always check whether the object is not \`null\` or \`undefined\`. This can be done simply with an if statement: \`if (obj) { console.log(obj.property); }\`.
Alternatively, consider using optional chaining (\`obj?.property\`) which safely returns \`undefined\` rather than throwing an error if \`obj\` is \`null\` or \`undefined\`.
These practices are crucial in dynamic environments where variables might not always be initialized as expected.
H7: Type checking for robust code
Finally, implementing type checks can fortify your code against unexpected types that lead to errors. Use JavaScript’s \`typeof\` operator to ensure the variable is of the expected type before performing operations on it.
For more complex type checks, or in case of relying on specific object shapes, consider integrating TypeScript or other static type checkers into your development workflow. These tools can help catch mistakes during the compiling process, well before the code runs.
Common Mistages Leading to Uncaught TypeError
Misuse of asynchronous functions
One typical source of "Uncaught TypeError: Cannot Read Properties of Undefined" in JavaScript involves improper usage of asynchronous functions.
Asynchronous functions, such as those that use promises or are marked with the async keyword, don’t complete immediately.
Developers might attempt to use the result of an asynchronous function that has not yet completed, leading to attempts to access properties of an undefined object.
For instance, if a function fetches data from an API and another function tries to execute using this data before it's fetched, a TypeError can occur if the data object remains undefined at the time of access.
Handling callback functions improperly
Callback functions are essential in JavaScript for handling sequences of operations, especially in asynchronous code execution. A common error stems from executing callbacks that assume certain data will be available, which might not be the case.
If the callback function tries to access properties of an input that hasn’t been properly initialized or returned, it will trigger an Uncaught TypeError.
This mistake often occurs in nested callbacks, where the error in the data flow can lead to mishandling of inputs and state management.
Lack of error handling in code
Often, developers overlook the necessity of implementing error handling mechanisms within their code. When functions are executed without checks on their expected inputs, any irregularities in those inputs can lead to runtime errors.
When an undefined object or a null value is accessed without prior validation, the JavaScript engine throws a TypeError.
Solutions to Uncaught TypeError
Proper error handling techniques
Implementing thorough error handling is a crucial step in safeguarding your JavaScript code against TypeErrors. This can include checking variables to ensure they are not undefined or null before attempting property access.
For instance, using conditional statements such as \`if (variable)\` before using \`variable.property\` can prevent TypeErrors by confirming the presence of a valid object.
Refactoring code for clarity and error prevention
Refactoring code can significantly enhance its readability and reduce the likelihood of errors. This involves simplifying complex functions, breaking large functions into smaller, manageable units, and using clear and consistent naming conventions for variables and functions.
Moreover, ensuring that each function is tasked with a single responsibility also helps in maintaining clarity, making it easier to spot potential errors and handle them appropriately.
Leveraging try...catch statements for exceptions
Utilizing try...catch statements in JavaScript provides a robust way to handle exceptions, including TypeErrors. By wrapping the suspect code block within a try statement and handling the error in the catch statement, you can manage the error gracefully without letting the entire application fail.
For example, if a portion of your code is prone to failing due to undefined properties, enclosing this portion in a try...catch block allows you to define a fallback operation in the catch clause, ensuring the application’s continuity.
Book a Demo and experience ContextQA testing platform in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
Understanding and addressing the Uncaught TypeError: Cannot Read Properties of Undefined is critical in JavaScript development. This common error can cause scripts to stop executing, leading to poor user experiences and software bugs.
By following the preventative tips and troubleshooting steps outlined, you can ensure more robust, error-free code. Remember always to check your object references, initialize your variables properly, and use debugging tools efficiently.
With these practices in place, maintaining and writing JavaScript code becomes more manageable and error-prone scenarios, like accessing properties on undefined, can be significantly reduced or even avoided.
Also Read - What Is an Emulator? Types, Top Benefits & Examples