Understanding the structure and organization of automated tests is crucial for enhancing efficacy and maintainability, particularly as the complexity of the application increases.
One popular method within Python for managing Web automation tests is through the implementation of Page Objects. This approach separates the test logic from the user interface (UI) details, placing element identifiers and layout details in separate classes.
By abstracting these elements, tests become more readable, robust, and easier to update. Page Objects serve as an intermediary between the actual tests and the elements on the web page, ensuring that changes in the UI require minimal changes in the test suite, essentially reducing the maintenance overhead and improving the scalability of test scripts.
Understanding Page Objects in Web Automation Testing
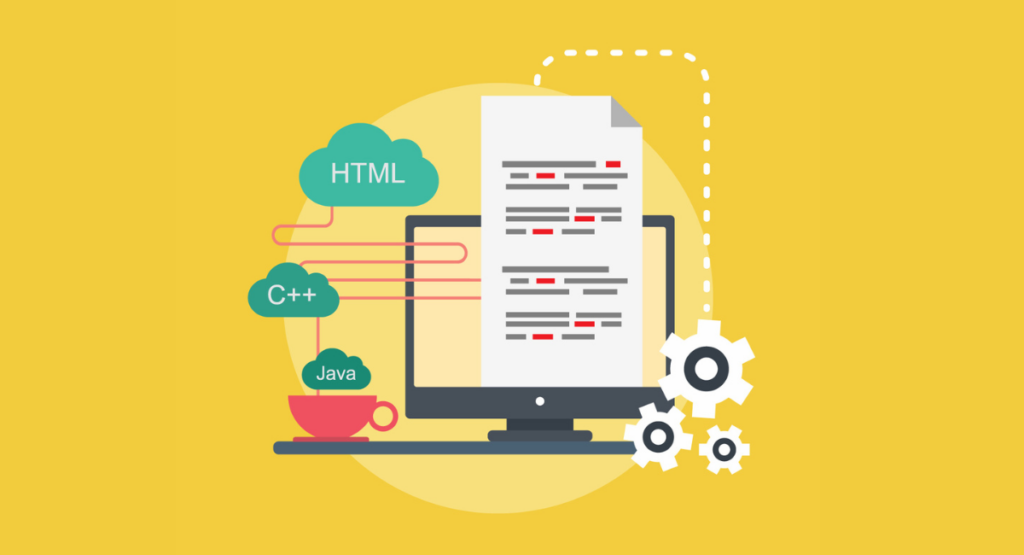
What are Page Objects?
One of the design patterns is Page Objects. It creates an abstraction layer between tests and the code controlling UI elements on the web page.
Again, every page in the system under test is represented by a corresponding class of Page Objects. This encapsulates direct UI element manipulation from the test scripts and makes the tests more readable and easier to maintain.
It structures the code that is interacting with an element on a single page, so changes to the structure of that page require updates only in the Page Object, not within tests themselves.
Benefits of Using Page Objects
Using Page Objects in testing provides several advantages:
- Maintainability: Changes in UI only need updates in Page Objects, not in all test scripts.
- Reusability: Functions defined in Page Objects can be used across different tests.
- Readability: Tests become more readable and understandable as technical details are hidden in Page Objects.
- Reduced Code Duplication: Common page elements and functionalities can be reused across multiple Page Objects and tests, minimizing code repetition.
Implementing Page Objects in Python
To implement a Page Object model in Python, you typically use a library like Selenium. Page Objects in Python are created as classes where each class represents a page on the web application.
Each class contains methods that interact with the elements of that specific page, providing an interface for the tests to interact with the page under test.
Getting Started with Selenium in Python
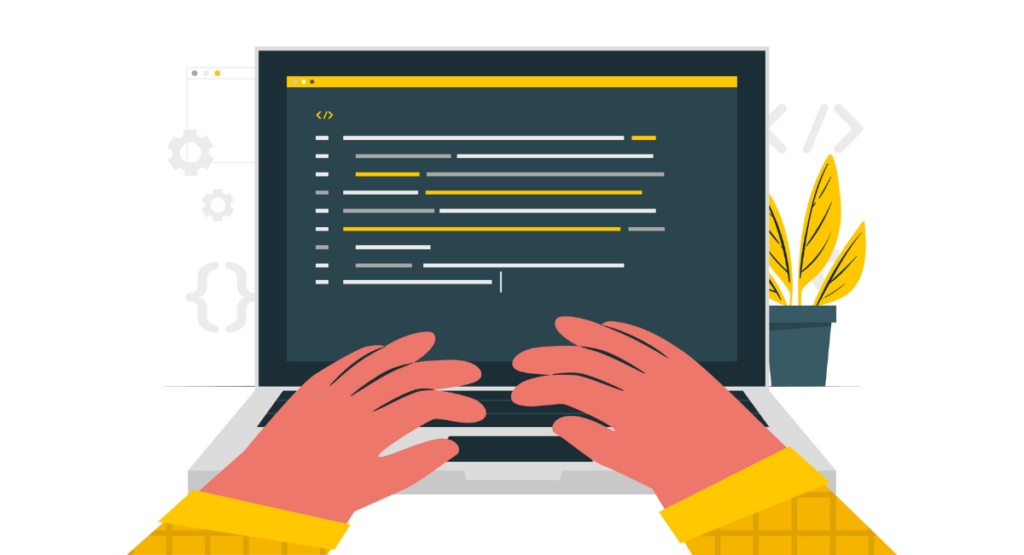
Installing Selenium
To use Selenium, you first need to install the \`selenium\` package. This is usually done with pip, Python's package manager. Just run \`pip install selenium\` in your command line and it will download and install the latest version of Selenium.
Setting up WebDriver for your browser
You will need an instance of WebDriver to be able to interact with the browser. Depending on the browser, you need to download the proper WebDriver executable, for example if you want to automate a test with Chrome or Firefox.
This executable needs to either be in a known location on your system, or referenced in your system's PATH environment variable. For example, if using Google Chrome, you would download ChromeDriver.
Creating your first Selenium test script
Once Selenium and WebDriver are set up, you can create a simple test script. Start by importing the necessary modules:
\`\`\`python
from selenium import webdriver
\`\`\`
Next, initiate a driver instance specifying the path to the WebDriver:
\`\`\`python
driver = webdriver.Chrome('/path/to/chromedriver')
\`\`\`
Then, direct the browser to open a webpage:
\`\`\`python
driver.get('http://www.example.com')
\`\`\`
Interact with elements:
\`\`\`python
element = driver.findelementby_id('some-id')
element.click()
\`\`\`
Finally, always remember to close the browser after your script runs:
\`\`\`python
driver.close()
\`\`\`
This basic script navigates to a website, performs an action, and then closes the browser. As you expand your testing, Page Objects can be integrated to manage these interactions more efficiently.
Developing Page Classes for Web Pages
Identifying Page Elements
In the development of page classes, the first thing that needs to be done to create this is the identification of all major page elements on a web page with which you are going to interact.
These may be buttons, text fields, drop-down menus, and any other interactive elements. Such elements may be located by tools like Selenium WebDriver in Python, given only HTML attributes like 'id', 'name', and 'class', or through XPath and CSS selectors. It's important to use unique and stable attributes to have reliable tests.
Creating Page Methods
Now that you have identified the elements, the next step is to define methods in your page classes for all the interactions and functionalities on the web page.
For instance, if you have a log-in page, you might be looking at methods like 'enterusername()', 'enterpassword()', and 'click_submit()'.
These methods will encapsulate the respective Selenium commands necessary to interact with the elements; this again keeps the tests easy to read and maintain.
Organizing Page Core Classes in a Hierarchical Structure
Organizing page classes in a hierarchical structure enhances code reusability and maintains clarity. Base classes can be created for common functionalities and element interactions shared across multiple pages.
Each page-specific class can then inherit from this base class, containing methods and elements unique to that particular page. This hierarchical model not master available in this message.
Handling Dynamic Web Elements with Page Objects
Dealing with Dynamic XPath
Dynamic web elements, whose attributes change with page reloads or due to other user actions, pose a challenge in automation. To handle these, dynamic XPath expressions are used.
These XPaths rely on aspects of the elements that do not change, such as a partially matching attribute value or a stable parent element. This approach allows the Page Object to flexibly locate elements under varying conditions, improving the robustness of your tests.
Implementing Wait Strategies for Dynamic Elements
Effective handling of dynamic elements also requires implementing wait strategies to ensure that elements are fully loaded or in the desired state before interactions.
Selenium WebDriver offers several waiting strategies, including explicit, implicit, and fluent waits. Using explicit waits is advisable as it allows specifying conditions that must be met within a maximum time frame, making the automation scripts more reliable and less prone to errors due to timing issues.
Implementing these strategies within your Page Objects helps maintain a clear and efficient testing codebase.
Best Practices for Page Object Model
Naming Conventions for Page Objects
When working with Page Objects, maintaining a consistent naming convention is crucial for enhancing readability and maintainability of your code. Class names should clearly reflect the page they represent, utilizing CamelCase notation.
For instance, if you are dealing with a home page, name the class HomePage. Similarly, methods within these classes should have descriptive names that reflect the action they perform, like findUserNameField() or submitLoginForm().
Adhering to these naming standards helps team members and future maintainers of the codebase understand the code more quickly and thoroughly.
Keeping Page Objects Separate from Test Logic
Separating page objects from test logic is fundamental to a clean and effective test suite. Page objects should solely be responsible for interacting with the UI elements of the web application, while the test scripts handle the flow of the test cases.
This separation allows you to reuse page objects across different tests and makes your tests easier to update when the UI changes. It also enhances the scalability of your test code by isolating changes to the page object layer without impacting the test logic.
Advancing Your Web Automation Testing with Page Objects
Extending Page Objects for Reusability
To maximize the efficiency of your testing framework, extend the functionality of basic Page Objects by creating base classes that can be inherited by more specific page classes.
This approach reduces the amount of duplicate code and eases maintenance. For example, if several pages on your site have a header with navigation links, you can create a BasePage class that includes common methods like navigateToHomePage() or search().
Other page classes can inherit from this BasePage, ensuring all common functionality is located in a single place.
Implementing Page Factories for Managing Page Objects
Page Factories are a design pattern used in conjunction with the Page Object Model to provide an optimized way to initialize the elements of the page you are testing.
In Python with Selenium, you can use the PageFactory class to automatically load page elements when the page objects are initialized. This feature simplifies the initialization of page objects and makes the code cleaner and more readable.
By using annotations to define properties and lazy initialization for web elements, Page Factories can help manage the lifecycle of these elements within the page objects, consequently, simplifying the codebase and enhancing test performance.
Troubleshooting and Debugging Page Objects in Python
Common Pitfalls in Page Object Model
When implementing Page Objects in Python for web automation, developers commonly face several challenges. One frequent pitfall is the lack of proper encapsulation which leads to repeated code and harder maintenance.
Another issue is not correctly handling dynamic elements, causing flakiness in tests. Developers might also construct overly complex page objects that attempt to cover too much functionality, making them cumbersome and difficult to manage.
Additionally, failure to synchronize properly with the web page's state can result in timing issues where commands are executed before the page is ready.
Tips for Debugging Page Objects
To effectively debug page objects in your test automation, consider the following strategies:
- Implement Explicit Waits: Utilize Selenium’s WebDriverWait class to ensure that elements are fully loaded before interaction.
- Use Logging Extensively: Incorporate logging at key points within your page objects to help trace the flow and diagnose issues.
- Break Down Complex Methods: If a page object method is too complex, break it down into simpler, reusable components. This makes it easier to pinpoint errors.
- Review Your Selectors: Regularly check and update your selectors. This is crucial as changes in the web application’s UI may lead to outdated selectors that can cause your tests to fail.
- Run Tests in Isolation: If a test fails, try running it in isolation to determine if the issue is with the test environment or the specific test case.
By adherently following these guidelines, you can mitigate common issues and enhance the stability and reliability of your page object models.
Book a Demo and experience ContextQA testing tool in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
In summary, implementing Page Objects in Python for your Selenium tests can significantly tidy up your testing suite, making scripts more readable, maintainable, and scalable.
By abstracting UI details into classes and methods, Page Objects allow you to write cleaner code that can be easily understood and updated, particularly useful in agile environments and for long-term projects.
Embrace Page Objects to elevate the robustness and clarity of your test automation efforts, ensuring that each test is reusable and resistant to changes in the web application's UI.
As Python continues to grow in its role within the testing community, utilizing its capabilities fully through patterns like Page Objects will surely place you at an advantage in the realm of web automation.
Also Read - What is Ad Hoc testing or monkey testing?
We make it easy to get started with the ContextQA tool: Start Free Trial.