The Python ConfigParser module, which is well-known for its simplicity and readability, provides an effective way to maintain configurations. This tool makes it easy for developers to write, read, and edit configuration file choices.
Configuration files are ideal for managing non-sensitive information, such as settings and preferences, helping to keep your code clean and maintaining a separation between code and data.
In this tutorial, we'll explore the capabilities of the ConfigParser library in Python, demonstrating how you can use it to manage your project configurations proficiently.
Whether you're new to Python or looking to streamline how you handle configurations in your applications, this guide will provide vital insights into effectively using the ConfigParser module.
Overview of Python ConfigParser
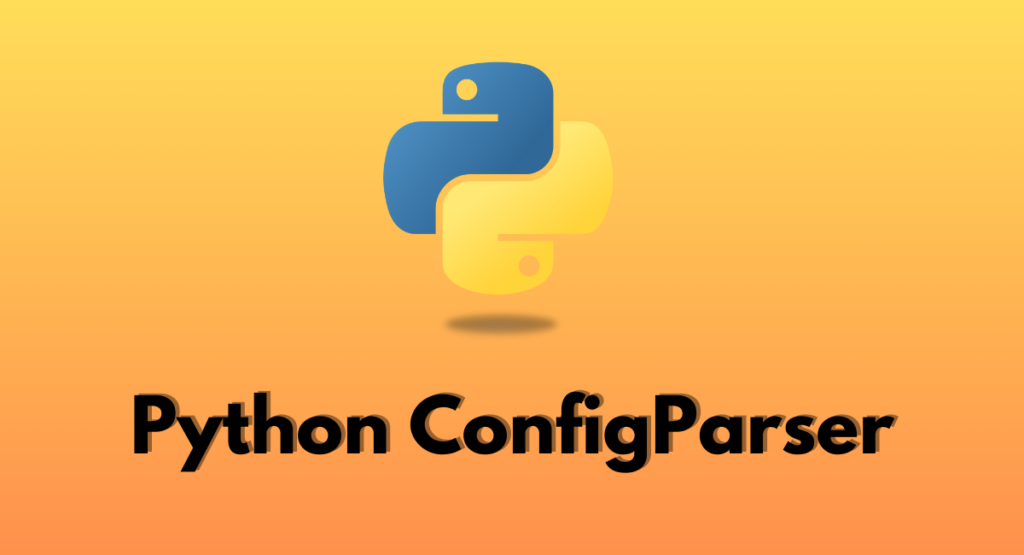
What is ConfigParser?
ConfigParser is a class provided by Python used to implement a basic configuration file parser. This library is part of Python’s standard utility modules and allows for the easy handling of configuration files.
Typical configuration files are written in a simple format comprising sections, keys, and values. Each section can contain a unique set of options or keys, and each key points to a respective value.
The ConfigParser module can read and write to these files, providing a programmatic way to access and modify the data.
Why use ConfigParser in Python?
The primary use of ConfigParser in Python is to enable applications to configure themselves without hard-coding information into the script.
Using configuration files makes your applications easier to deploy in different environments, simplifies complex system configurations, and enhances overall code manageability and scalability.
Furthermore, it allows non-programmers such as system administrators to adjust application settings without diving into the codebase, making ConfigParser an essential tool for developing robust, user-friendly software.
Basic Operations with ConfigParser
Installing ConfigParser
Before using ConfigParser, you need to ensure that it is installed on your system. Although ConfigParser is included with Python’s standard utility modules, in some cases, such as when working in a virtual environment, you might need to install it manually using Pip. You can install ConfigParser by running the following command in your terminal:
\`\`\`bash
pip install configparser
\`\`\`
This command will download and install the ConfigParser package, allowing you to import it into your Python scripts.
Reading configuration files
To read from a configuration file using ConfigParser, you must first import the module, create an instance of ConfigParser, and load the configuration file by calling the \`read\` method.
Here’s a step-by-substep guide on how to perform these operations:
- Import the ConfigParser module:
\`\`\`python
import configparser
\`\`\`
- Create an instance of ConfigParser:
\`\`\`python
config = configparser.ConfigParser()
\`\`\`
- Load the configuration file:
\`\`\`python
config.read('example.ini')
\`\`\`
Once loaded, you can access the configuration settings easily. For example, if you want to get a specific configuration value:
\`\`\`python
host = config['DEFAULT']['ServerHost']
\`\`\`
This operation will retrieve the 'ServerHost' value from the 'DEFAULT' section of the 'example far.ini' file.
Writing to configuration files
Writing data to a configuration file involves setting the values of parameters within sections of your config file. Here are the steps to write to a configuration file using ConfigParser:
- Define a new section or select an existing one:
\`\`\`python
config['DEFAULT'] = {'ServerHost': 'localhost'}
\`\`\`
- Assign new options and values in the section:
\`\`\`python
config['DEFAULT']['User'] = 'admin'
\`\`\`
- Write changes back to a file by opening the file in write mode:
\`\`\`python
with open('example_updated.ini', 'w') as configfile:
config.write(configfile)
\`\`\`
This example shows how to modify the 'DEFAULT' section by changing the 'ServerHost' to 'localhost' and adding a new 'User' key with the value 'admin'.
The final step involves writing the modified data back to a new or the same configuration file to save these changes. Through these basic operations, ConfigParser provides a full suite of functionalities for managing configuration files effectively.
Advanced Operations with ConfigParser
Updating settings
Updating settings in Python using the ConfigParser library involves reading the configuration file, modifying the values, and then writing them back to the file.
To do this, first load the configuration using \`config.read('filename.ini')\`. Then, use the \`set\` method to change the value in the configuration. For instance, \`config.set('Section', 'option', 'new value')\`.
After modifying the settings, save the changes back to the file with \`with open('filename.ini', 'w') as configfile: config.write(configfile)\`. This process is essential for applications where configuration needs to reflect changes dynamically.
Error handling with ConfigParser
Effective error handling in ConfigParser is crucial to develop robust applications. Common errors include missing sections or options, and issues reading or writing to files. Use try-except blocks to catch these exceptions gracefully. For example:
\`\`\`python
try:
config.read('filename.ini')
print(config['DEFAULT']['Option'])
except KeyError:
print("Section or option is missing")
except Exception as e:
print(f"An error occurred: {e}")
\`\`\`
This approach ensures that your program can handle unexpected issues without crashing and provides informative feedback to the user.
Adding comments to configuration files
Comments in configuration files help maintain clarity about the purpose and function of each option. When using ConfigParser, comments can be included directly in the INI file by prefixing the line with a semicolon (\`;\`) or a hash (\`#\`). These comments will not be parsed by the ConfigParser and will remain when the file is read. For instance:
\`\`\`ini
# This is a comment
[Section]
option = value ; another comment
\`\`\`
Including explanatory comments in configuration files can greatly enhance the maintainability and readability of code, especially in a collaborative setting.
Best Practices for Working with ConfigParser
Organizing configuration files
Proper organization of configuration files is crucial for maintainability and scalability. Adhere to a logical structure that separates different aspects of the application.
For complex applications, consider splitting the config into multiple sections or even separate files based on functionality (like database settings, application settings, etc.).
Always aim for clarity, using descriptive names for sections and options to make the configuration self-documenting as much as possible.
Handling sensitive information
Sensitive information such as passwords, API keys, or secret tokens should never be hard-coded into your configuration files. Instead, consider using environment variables or encrypted secrets management services.
Libraries like \`os\` in Python can be used to read environment variables: \`password = os.getenv('DATABASE_PASSWORD')\`. This method enhances the security of your application by separating sensitive credentials from your codebase.
Version control for configuration files
While it may be tempting to include configuration files in version control, it's vital to treat them with care, especially files containing sensitive information.
For generic configurations, include a sample configuration file (e.g., \`config.ini.example\`) in the repository with generic values or placeholders.
Ensure that actual configuration files with sensitive or critical information are excluded from version control through \`.gitignore\` or similar mechanisms.
This practice prevents sensitive data from being exposed and helps maintain different configurations for development, testing, and production environments without conflict.
Book a Demo and experience ContextQA testing platform in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
In this tutorial, we have thoroughly explored the functionality and application of the ConfigParser module in Python for handling configuration files efficiently.
Whether storing program settings, managing user options, or facilitating data interchange between various parts of a complex system, ConfigParser provides a robust, readable, and writable solution that integrates smoothly with the Python language.
By mastering ConfigUation files through Python ConfigParser, you can significantly streamline your coding practice, ensuring that your projects are not only functional but also well-organized and scalable.
Embrace the flexibility and power of configuration files to make your Python programs more dynamic and user-responsive.
Also Read - How To Use XPath in Selenium: Complete Guide With Examples