Selenium is among the robust sets of tools for web automation, letting developers and testers simulate a myriad of interactions by users with most of the available web browsers.
Google Chrome is one of the most used browsers while running Selenium tests, which involves using ChromeDriver.
ChromeDriver acts as a sort of intermediary or bridge between the Selenium tests and the Chrome browser that interprets different kinds of commands and executes them accordingly.
Such a setup gives ultimative control over the browser and thus allows test scenarios to simulate the activities of a real user.
Mastering how to configure and run Selenium tests with ChromeDriver in Chrome will save your time while providing for a more efficient and effective quality assurance.
Setting Up Selenium Tests on Chrome using ChromeDriver
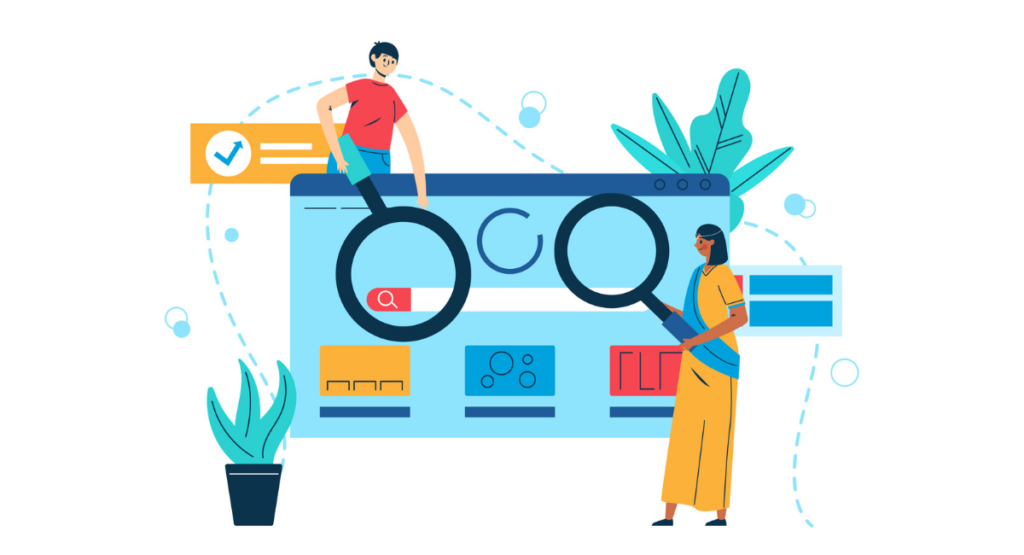
Installing ChromeDriver
First of all, you will have to download and install ChromeDriver. It is a standalone server which implements WebDriver's wire protocol for Chromium.
You can download the matching version according to your Chrome browser version from the page: ChromeDriver download page.
You can easily download ChromeDriver from ChromeDriver download page. After downloading, extract the executable into a known directory on your system.
It's important to note that this ChromeDriver should be put in a directory included in your system's PATH environment variable, or the location will have to be explictly referenced from code, which we cover a little later in Configuring the Selenium WebDriver.
Configuring Selenium WebDriver for Chrome
After ChromeDriver is installed, you need to configure Selenium WebDriver to make use of ChromeDriver. You do this by setting the path to the ChromeDriver executable in your test script. Here is how you would do this in a Java environment:
\`\`\`java
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
WebDriver driver = new ChromeDriver();
\`\`\`
In this code snippet, replace “/path/to/chromedriver” with the actual path to your ChromeDriver executable. This step informs Selenium which driver to use for Chrome browsers and initializes a new instance of ChromeDriver.
Handling Chrome Options
Chrome Options allow you to set various properties on your Chrome browser at runtime. You can customize your testing environment by adjusting these options.
Here are a few common configurations using Chrome Options:
- Headless mode: Running Chrome without a GUI.
- Disable extensions: Running Chrome without any extensions.
- Set binary path: Specifying the path to the Chrome binary.
Here’s how you can configure these options:
\`\`\`java
ChromeOptions options = new Chrome para[element was missing];
Writing Your First Selenium Test on Chrome
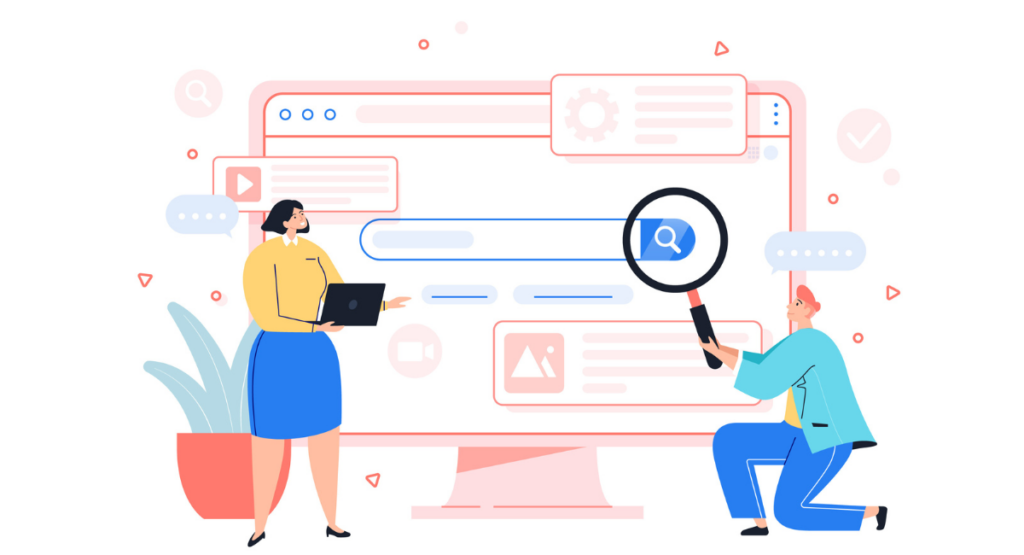
Opening a URL
The first step in creating a Selenium test is to open a URL. This is done using the \`get()\` method of the WebDriver instance. Here is an example using the previously defined \`driver\`:
\`\`\`java
driver.get("https://www.example.com");
\`\`\`
This code will open the Chrome browser and navigate it to the provided URL. It is the basis for all operations on the webpage.
Locating Elements
You will need to locate any web elements before taking any action on them. The different strategies for locating elements in Selenium are ID, Name, Class Name, Tag Name, CSS Selector, and XPath. Ways of locating an element using its ID is as shown below:
\`\`\`java
WebElement element = driver.findElement(By.id("element_id"));
\`\`\`
Replace "element_id" with the actual ID of the web element you want to access. Proper choice of a locator is crucial for Selenium to successfully and reliably find elements in your tests.
Interacting with Elements
Now that you've successfully located elements, most of the time you'll want to do something with them. You'll want to click, type text in text fields and select from dropdowns.
Here's an example of typing text in a text field. It uses the \`sendKeys()\` method:
\`\`\`java
element.sendKeys("Hello, Selenium!");
\`\`\`
To click a button, use the \`click()\` method:
\`\`\`java
driver.findElement(By.id("submit_button")).click();
\`\`\`
These methods allow you to simulate user actions and test how the web page behaves in response to those actions.
Advanced Selenium Test Techniques for Chrome
Waiting Strategies
Efficiency in automated tests isn’t just about speed but also about stability and reliability. Implementing smart waiting strategies in Selenium can significantly enhance the robustness of your test scripts. Selenium WebDriver offers several waiting mechanisms:
- Implicit Wait: Automatically waits for a specific amount of time before throwing an exception if it cannot find an element. This is a global setting applicable to all elements.
- Explicit Wait: Waits for a certain condition to occur before proceeding with the execution. This is useful for handling scenarios where certain elements take longer to load.
- Fluent Wait: Involves polling the DOM at regular intervals until a timeout or until the desired element is found. It allows you to specify what conditions to check and what exceptions to ignore, providing maximum control over the wait conditions.
These techniques help manage timing issues between the browser and your test, reducing the likelihood of encounteringElementNotVisibleException or TimeoutException during test runs.
Handling Alerts and Pop-ups
Alerts and pop-ups can be disruptive to the flow of an automated test, but Selenium WebDriver provides methods to handle such scenarios:
- switchTo().alert(): This method switches the context to the current alert on the page.
- accept(): Used for clicking 'OK' or accepting the alert.
- dismiss(): Used for clicking 'Cancel' or dismissing the alert.
- getText(): Captures the text from the alert box, which can be useful for validation purposes.
- sendKeys(): Sends a string to the alert box, typically used for providing input in prompt alerts.
Understanding and utilizing these methods will enable your Selenium tests to interact seamlessly with alerts and pop-ups in Chrome.
Working with Frames and Windows
Complex web applications often use frames or multiple windows which can pose a challenge for Selenium testing. To handle these elements:
- switchTo().frame(): You have to switch the WebDriver’s context to the relevant frame to interact with elements within that frame.
- switchOrwindow(): This method is crucial when dealing with multiple windows. It allows the WebDriver to switch between different windows based on window handles.
Mastering these commands ensures your tests are not obstructed by structural complexities of the web application.
Best Practices for Running Selenium Tests on Chrome
Test Organization
Organizing tests clearly is crucial for maintainability and scalability. Adhere to best practices like using Page Object Model (POM), which helps in organizing the code related to web pages separately as objects.
This can simplify the maintenance of your code base, as changes in the UI can be made with minimal adjustments in the test scripts.
Test Data Handling
Effective test data management helps in executing automated tests smoothly:
- Externalize Test Data: Store test data outside of your script, such as in XML files, JSON files, or databases. It keeps your tests clean and makes it easy to update the data without touching the code.
- Data-Driven Testing: Utilize frameworks like TestNG or JUnit to run your tests with different sets of data. This confirms that your application can handle various input scenarios smoothly.
Reporting and Logging
Accurate reporting and logging are essential for understanding the health of your test suite:
- Logs: Implement logging within your tests to record actions, decisions, and errors. Tools such as Log4j or SLF4J can be utilized for this purpose.
- Reports: Use tools like ExtentReports or Allure Reports to generate rich HTML reports for test executions. These can include detailed logs, screenshots on failure, and metrics such as pass/fail rates which are crucial for continuous integration workflows.
Following these best practices not only helps in reducing test failures due to operational inefficiencies but also enhances the clarity and accuracy of test results, making your Selenium tests on Chrome more effective.
Troubleshooting Common Issues in Selenium Tests on Chrome
Stale Element Reference Exception
If you receive a Stale Element Reference Exception in Selenium, it typically means either that the web element you're trying to interact with has changed since you've located it or the page has refreshed. Now, to resolve that problem:
- Make sure that the whole page is loaded before interacting with its elements. Explicit waits are applicable here; they will wait for certain conditions, for example, for the visibility of an element, and then proceed.
- Re-locate the web element right before you interact with it. This can ensure that you are working with the current instance of the DOM element.
- Check if any AJAX calls or dynamic content updates might be causing the element to reload or change state, and adjust your test logic to accommodate these changes.
Element NotTask Visible Exception
The Element Not Visible Exception typically occurs when an element is present on the DOM but not visible on the screen. This can be due to various reasons like element being obscured by other elements or because it is outside the viewport. To handle this:
- Scroll to the element before performing any action on it. You can achieve this with JavaScript (\`window.scrollTo\` function) or by using Selenium’s built-in functions like \`scrollIntoView\`.
- Use explicit waits with conditions like \`visibilityOfElementLocated\` to ensure the element is visible and interactable before trying to interact with it.
- Consider any CSS styles (like display settings) or JavaScript that might be hiding the element temporarily, and wait for those conditions to change if necessary.
Slow Element Interactions
Slow element interactions can frustrate the purpose of automation and increase test execution times. To speed up interactions with web elements in Chrome, consider the following tips:
- Use faster locators such as ID or class over XPath, as XPath tends to be slower especially in complex DOM structures.
- Adjust the pageLoad and script timeouts based on network speed and page size. This helps manage the time Selenium waits for a page to be considered fully loaded.
- Enable the ‘dom.webcomponents.enabled’ preference in your Chrome settings if your application uses Shadow DOM elements, which can also impact performance.
- Verify there is no excessive logging by Selenium or the application itself as it can significantly degrade the performance.
These adjustments can help streamline your Selenium tests, making them both faster and more reliable when running on Chrome with Chrome stringByAppending SerializableInfo
Book a Demo and experience ContextQA testing tool in action with a complimentary, no-obligation session tailored to your business needs.
Conclusion
Running Selenium tests using ChromeDriver is a powerful approach to ensure the robustness and functionality of web applications. Mastering this tool can enhance your testing capabilities and streamline your development workflow.
Remember, the key to effective testing lies in detailed planning, writing comprehensive test scripts, and regularly updating the tools and drivers as per browser updates.
By following the steps outlined in this guide, you can efficiently execute automated tests and significantly improve the testing process, contributing to the overall quality of your software products.
Stay updated with the latest releases from both Selenium and Chrome to keep your testing environment effective and efficient.
Also Read - Angular vs React vs Vue: Core Differences
We make it easy to get started with the ContextQA tool: Start Free Trial.